The “$?
” is a built-in variable that your shell uses to store the exit status code of the last executed command in integer format and remains unchanged unless the next command is executed.
Using this exit status code, you can debug the problem that occurred while executing the command, which can be extremely beneficial in shell script error handling.
The following is the list of known exit status codes from total (0-255
) for bash in Linux:
Exit status | Description |
---|---|
0 | If no command is executed or a command is successfully executed, its status is set to zero. |
1 | It will catch the general errors. |
2 | It will catch the shell built-in errors. |
126 | Commands invoked cannot be executed. |
127 | Command not found. |
128 | Invalid argument to exit. |
128+n | Fatal error signal “n”. |
130 | The script was terminated by “Control+C“. |
255 | The exit status is out of range. |
Checking the Bash Exit Status Code
The following is a demonstration of the known bash exit status code:
Example of Exit Status Code “0”
By default, when you start or restart your system (or shell session) without executing any commands, the exit status code is set to zero.
$ echo $?
Output:

Even if you execute any command with successful output, the exit status will remain zero.
$ pwd
$ echo $?
Output:

Example of Exit Status Code “1”
If you pass a wrong or invalid option to the command, it will set the exit status code to a non-zero value.
$ cat -a file.txt
$ echo $?
Output:
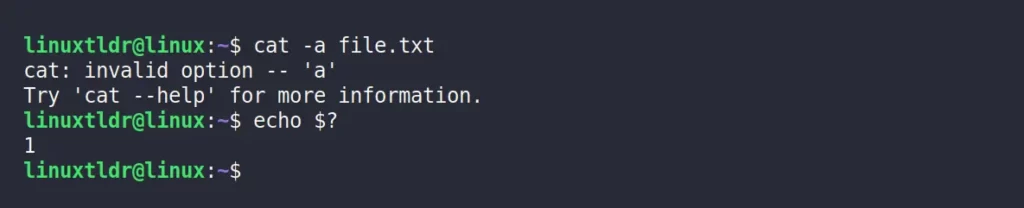
The above exit status code “1
” represents that the last executed command provided an invalid option.
Example of Exit Status Code “2”
If you pass a wrong or invalid argument to the command, it will set the exit status code to “2
“.
$ ls wrongfile
$ echo $?
Output:

Example of Exit Status Code “127”
If you mistyped or executed the wrong command, it will set the exit status code to “127
“.
$ wrongcommand
$ echo $?
Output:

Example of True or False Keywords
Even if you execute keywords like “true
“, it will set the exit status code to “0
“, and for “false
“, the value will be set to “1
“, making it highly effective while writing a shell script.
$ true
$ echo $?
$ false
$ echo $?
Output:
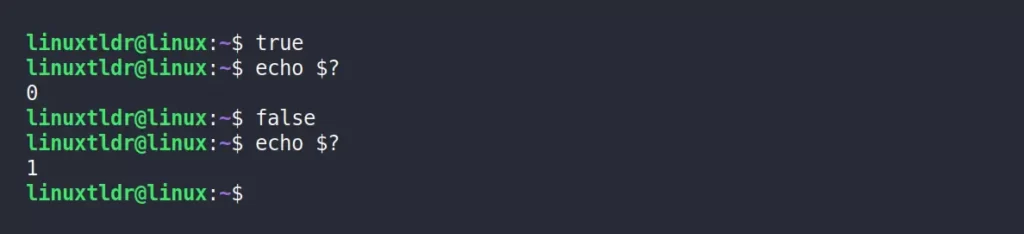
Checking the Exit Status Code of Piped Commands
If you have piped one or more commands together, the exit status will store the value of the last executed command.
For the following piped commands, it will show you the exit status code for the PWD command.
$ WRONGCOMMAND | pwd
$ echo $?
Output:
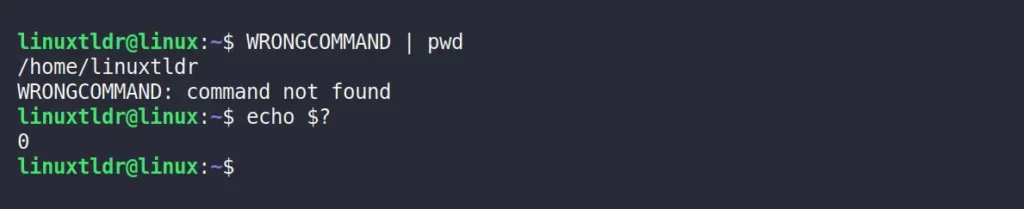
Controlling the Flow of Script Using the Exit Status Code
The exit status code can be extremely helpful while writing shell scripts to handle the flow of your script with all possible errors.
For example, the following is a basic demonstration of handling a script using the exit status code:
#!/bin/bash
echo "Its, Linux TLDR"
status=$?
[ $status -eq 0 ] && echo "command succeeded" || echo "command failed"
If you run the above script, it will print “command succeeded
” on an exit status code of “0
” and “command failed
” on a non-zero exit status code.
$ ./script.sh
Output:

However, if you modify the same script and misspell or include a wrong command, as shown.
#!/bin/bash
WRONG-COMMAND
status=$?
[ $status -eq 0 ] && echo "command succeeded" || echo "command failed"
It will print “command failed
” rather than “command succeeded
“.
$ ./script.sh
Output:

The Meaning of the Exit Status Code Value Might Be Different for Different Commands
Most of the commands, like echo or cat, follow the standard exit status code. However, the meaning of the exit status code value might vary for other commands like ls and grep.
Both commands have different explanations for exit status codes “1
” and “2
“.
The following is the exit status code of the ls command.

The following is the exit status code of the grep command.

So, before assigning the condition using the exit status code of commands, I strongly recommend that you check their manual page or search for the command on our site (most probably you will find it) to find the meaning of their default exit status code.
If you are too lazy to do that, then define your own exit status code.
Defining the Custom Exit Status Code in Script
To remove the headache of different commands having different meanings for exit status codes, you can manually specify your own custom exit status code for a specified command (or condition) using the exit keyword.
As per rule, keep the “0
” value reserved for “successful execution
” and use the value between the range of (1-255
) for errors.
The following is a basic script that will check if the current user is root or not; if the user is root, it will print “Root user
” on screen with an exit status code of “0
” or “Not root user
” with an exit status code of “1
“.
#!/bin/bash
if [[ "$(whoami)" == "root" ]]
then
echo "Root user"
exit 0
else
echo "Not root user"
exit 1
fi
Executing the above script with and without the root user.
#Executing as a non-root user
$ ./script.sh
$ echo $?
#Executing as a root user
$ sudo ./script.sh
$ echo $?
Output:
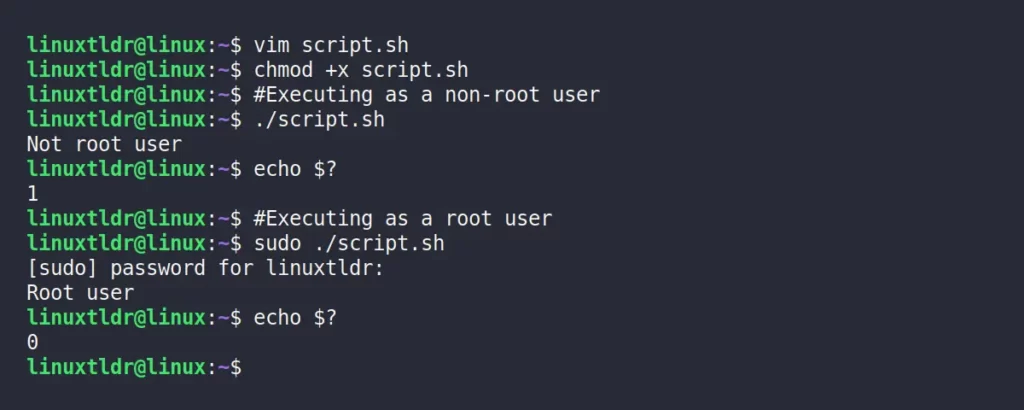
And that was the end of this guide.
If you have any suggestions or good examples that need to be added to this article, please share them with us in the comment section.
Sayonara!
### Comment:
This article provides an excellent and detailed explanation of the importance and usage of the “$?” exit status code in Linux. Understanding these exit codes is crucial for effective error handling and debugging in shell scripting. The practical examples and explanations for various exit status codes (0, 1, 2, 127, etc.) are particularly helpful for both beginners and experienced users. The guide on controlling script flow using these codes, as well as defining custom exit status codes, adds significant value. Overall, this is a must-read for anyone looking to enhance their shell scripting skills and ensure robust script execution in
Linux.
For better understanding, here are the some excellent learning platforms.
1.https://www.w3schools.com/
2. https://iqratechnology.com/
3. https://www.javatpoint.com/