The set command is a built-in Linux command that can display or modify the value of shell attributes and positional parameters inside the current shell environment.
This modification can help to debug your script by finding undefined variables, errors, job control, printing commands as they are executed, and automatically exporting variables and functions in sub-shell.
Tutorial Details
Description | Set |
Difficulty Level | Low |
Root or Sudo Privileges | No |
OS Compatibility | Ubuntu, Manjaro, Fedora, etc. |
Prerequisites | set |
Internet Required | No |
Syntax of the Set Command
The set command takes two arguments: one is the option, and the other is the argument.
$ set [OPTION] [ARGUMENT]
Understanding the [OPTION]
The “option
” is used to set or unset attributes or parameters in the current shell environment, affecting the behavior of your scripts and helping execute the desired tasks.
The following is a list of the most relevant options used with the set command.
-
“) with a plus sign (“+
“) followed by the appropriate option.Options | Description |
---|---|
-a | Mark variables that are modified or created for export. |
-b | Alert the user on background job termination. |
-e | Once the shell receives a non-zero exit status, it will terminate the script execution immediately. |
-f | Disable file name generation (globbing). |
-h | Enable saving the commands by default as they are looked up. |
-k | All assignment arguments are placed in the environment for a command, not just those that precede the command name. |
-m | Display a message when a task completes. |
-n | Monitor commands but not execute them. |
-p | Disable the processing of the “$ENV ” file and the import of shell when the real and effective user ids do not match. |
-t | Exit immediately after reading the first command. |
-u | Treat unset or undefined variables as an error when substituting, except for special parameters like wildcard. |
-v | Print out shell input lines while reading them. |
-x | Print commands with their arguments as they are executing. |
-C | If set, disallow existing regular files to be overwritten by the redirection of output. |
Understanding the [ARGUMENT]
The “argument
” is a positional parameter or variable that holds a value that can be used in a shell script.
The positional parameter will reference the position of the value as “${N}
” in order, where “N
” denotes the position of the parameter.
$1
$2
...
$n
For example, “$1
” is the first positional parameter after the command. The “$2
” value is the second parameter, and so on.
Exit Status
The exit status comes in handy when using the set command within your script to manage the flow of your shell script.
The set command has three exit statuses:
0
: Successful.1
: Failure caused by an incorrect argument2
: Failure is caused when an expected argument is missing.
Using the Set Command Without Options
Running the set command without an argument or options will print the long list of shell variables with their names and values.
$ set
Output:
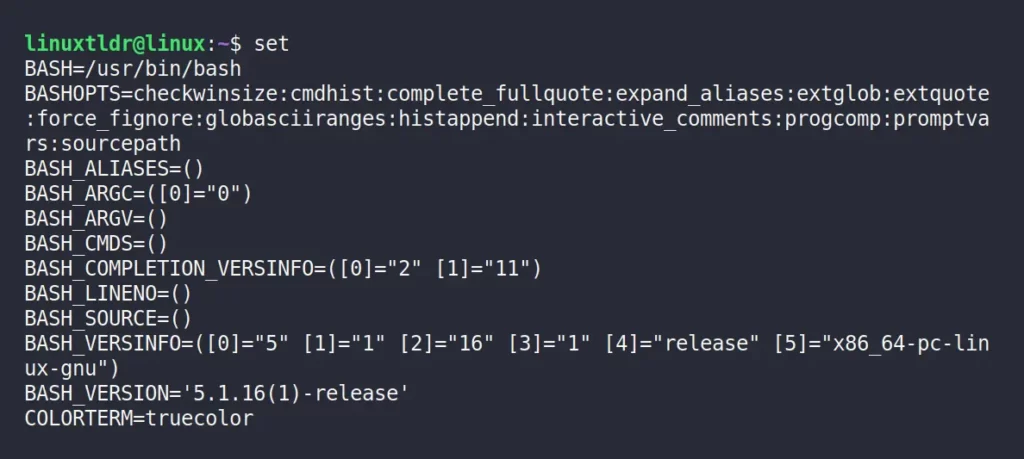
The list will be too long, so you can pipe the command with the “less
” keyword to enable interactive reading.
$ set | less
Output:
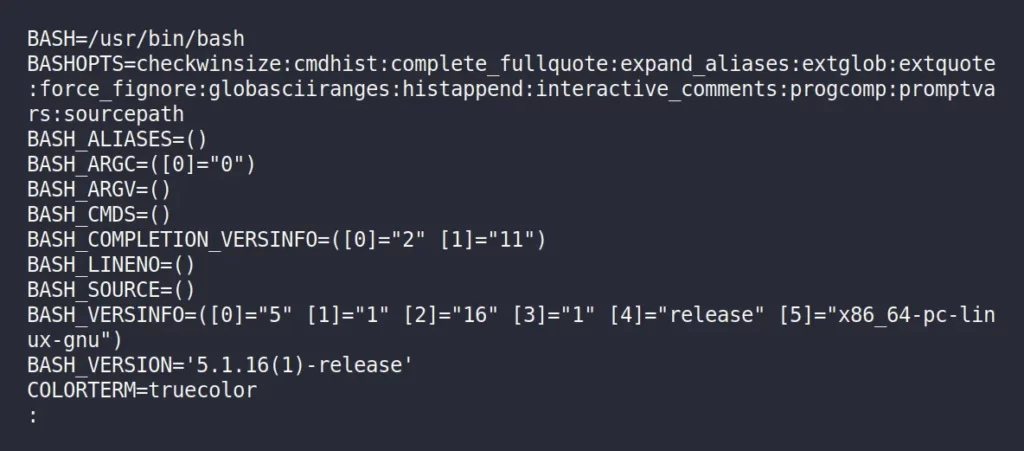
Turn Script Debugging Information On or Off
The “-x
” flag is used to turn on script debugging information that will print each command as they are executed in the output to help you understand the behavior of your script.
To understand the usage of this option, create a file with the name “script.sh
” and copy the following content into it.
echo "Hi"
echo "its"
echo "Linux"
echo "TLDR"
To run your shell script, give it the necessary executable permission using the chmod command.
$ chmod u+x script.sh
Now, before executing the script, enable debugging using the “-x
” flag.
$ set -x
Execute your shell script.
$ ./script.sh
Output:
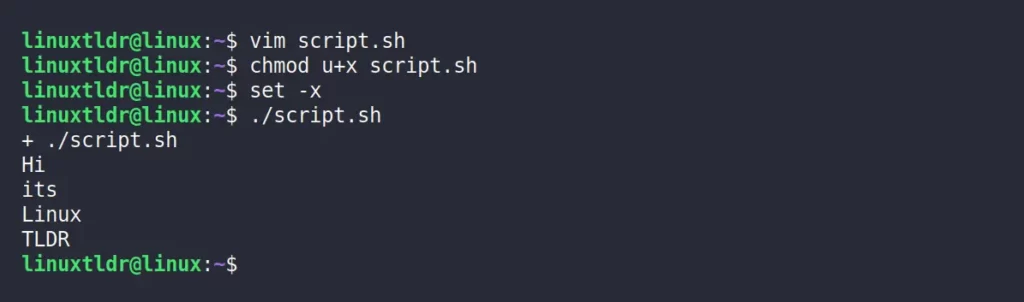
This option will print the commands in the sequence as they are executed, moving each step one by one.
Another way to use this option within your script is by specifying the “-x
” flag on the shebang line.
#! /bin/bash -x
Execute the following command with the plus sign “+
” to turn off script debugging information.
$ set +x
$ ./script.sh
Output:
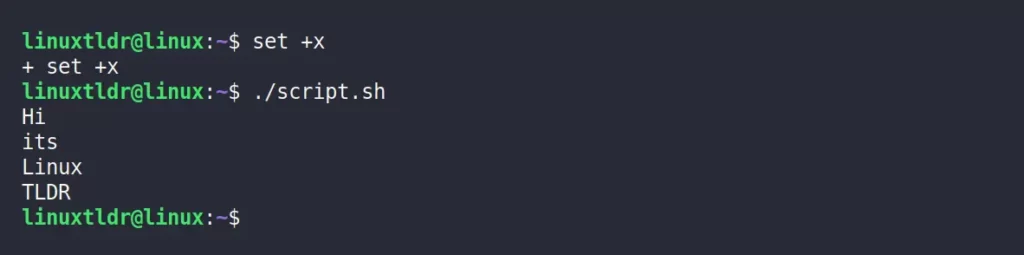
Setting Positional Parameters With the Set Command
Directly specify the values as an argument to the set command with a space to reference each value as “${N}
” in order, where “N
” denotes the position of the parameter.
$ set apple banana cat dog
$ echo $1
$ echo $2
$ echo $3
$ echo $4
Output:
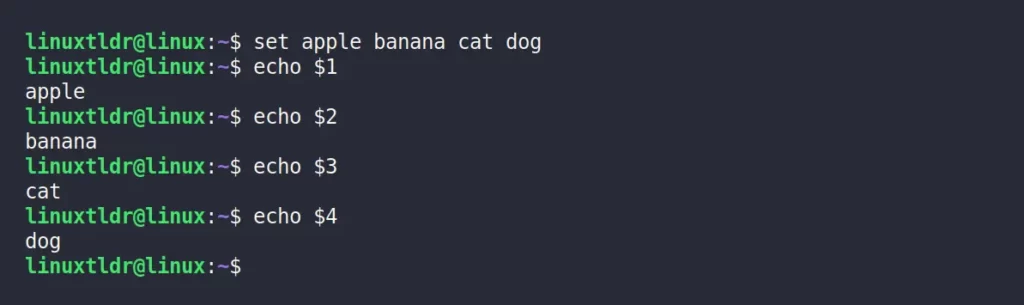
Execute the echo command with “$*
” to print the values of all parameters.
$ echo $*
Output:

You can even split the variable values based on spaces using the “set -- [VARIABLE]
” command.
$ fruit="apple banana cat dog"
$ set -- $fruit
$ echo $1
$ echo $2
$ echo $3
$ echo $4
Output:
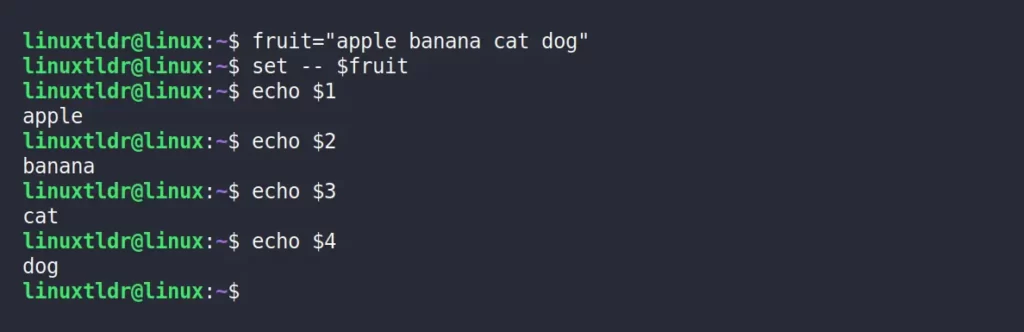
Use the “--
” with the set command to remove the assigned values from the positional parameters.
$ set --
$ echo $*
Output:

Exporting Variables or Functions to a Sub-Shell
By default, whenever you can create variables or functions, they are only accessible from the parent shell in which they were created.
However, you can use the “-a
” flag to export the variables or functions inside the sub-shell.
Without flag:
$ site=linuxtldr.com
$ bash
$ echo $site
Outptu:

With flag:
$ set -a
$ site=linuxtldr.com
$ bash
$ echo $site
Output:
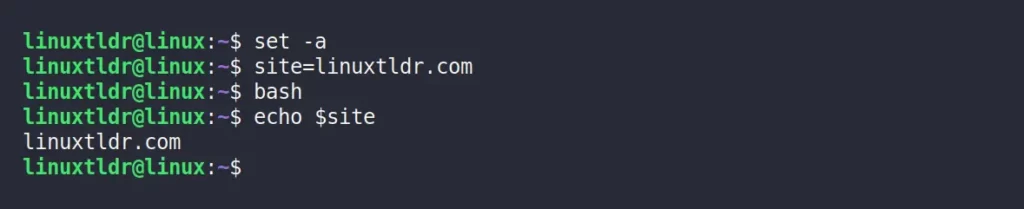
Immediately Exit When Command Fails
During the script execution, if it encounters any errors, it will print the error on the screen and keep executing the rest of the commands in the script.
The following is the script that includes an error.
echo "Hi"
its
echo "Trend"
echo "Oceans"
The following is an example of executing the script without any error control:
$ ./script.sh
Output:
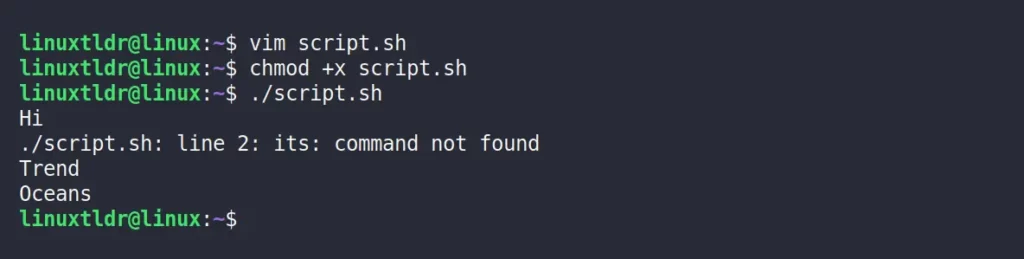
As you can see, the script kept executing even after the error.
To prevent this behavior of script execution, use the “-e
” flag to prevent the script immediately after it encounters its first error.
The following is the previous script with error control.
set -e
echo "Hi"
its
echo "Trend"
echo "Oceans"
The following is an example of executing the above script with error control:
$ ./script.sh
Output:
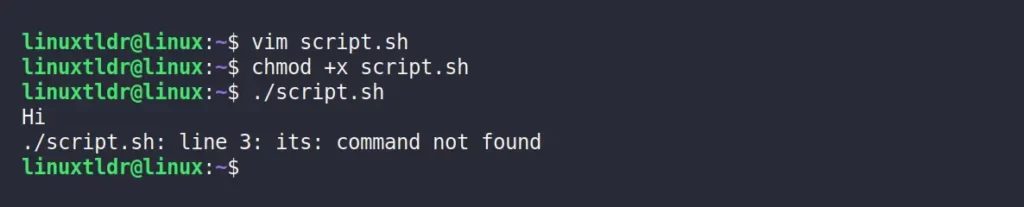
Note that this method will not prevent error control during pipe execution.
For example, the following is the same script, but an error is piped with the echo command.
set -e
echo "Hi"
wrong-command | echo "its"
echo "Trend"
echo "Oceans"
If you run the above script even with the “-e
” flag, it will keep executing after it encounters an error.
$ ./script.sh
Output:
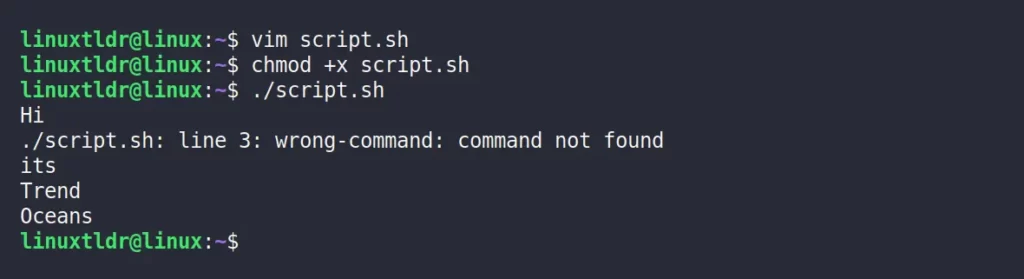
Use “set -eo pipefail
” instead of “set -e
” to overcome this problem.
set -eo pipefail
echo "Hi"
wrong-command | echo "its"
echo "Trend"
echo "Oceans"
Executing the script.
$ ./script.sh
Output:
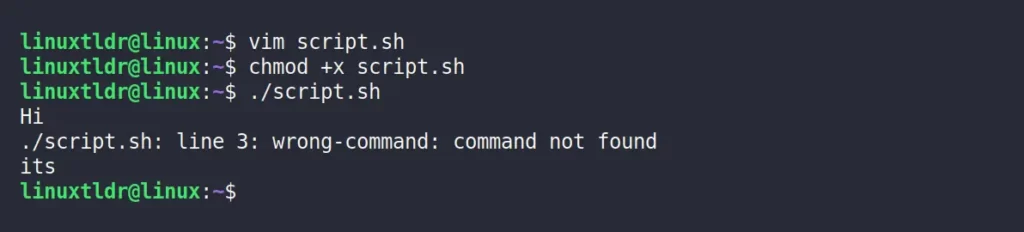
Prevent File Overwriting using the Redirection Symbol
The default setting in Bash is to keep overwriting the specified file using the “>
“, “>&
“, or “<>
” redirection symbols. However, you can overcome this problem using the “-C
” flag.
$ echo "Overwriting" > file.txt #File overwritten
$ echo "Overwriting" > file.txt #File again overwritten
$ set -C #Disallowing file from being overwriting
$ echo "Overwriting" > file.txt #Unable to overwrite the file
Output:

The above file can still be overwritten using the “>>
” redirection symbol.
$ echo "Overwriting" > file.txt
$ echo "Overwriting" >> file.txt
Output:

Restrict Undefined Variables
Whenever you execute the script file while calling undefined or unbound variables, the script keeps executing without throwing any errors.
The following is the script calling an undefined variable.
echo "Welcome"
$st
echo "Bye"
Even if the value of the variable “$st
” is not assigned, the script will keep executing.
$ ./script.sh
Output:

Use the “set -u
” command at the top of the script to restrict the execution of the script after it encounters the first unbound variable during execution.
set -u
echo "Welcome"
$st
echo "Bye"
Executing the script:
$ ./script.sh
Output:
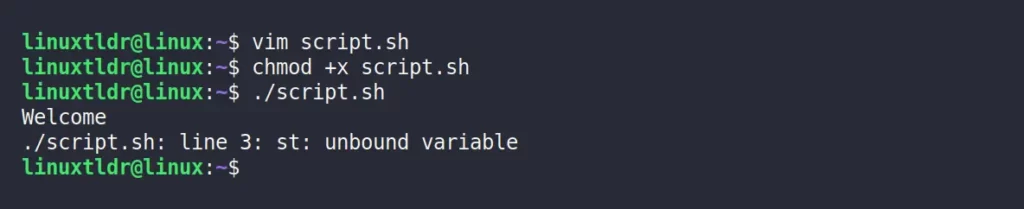
Set Allexport and Notify Flags
Use the “-o allexport
” flag to automatically export all subsequently defined variables, with the “-o notify
” flag to print the job completion messages.
$ set -o allexport -o notify
$ rm script.sh &
Output:

And that was the end of this article.
If you have any suggestions or questions related to this topic, feel free to ask them in the comment section.
Join The Conversation
Users are always welcome to leave comments about the articles, whether they are questions, comments, constructive criticism, old information, or notices of typos. Please keep in mind that all comments are moderated according to our comment policy.