Python is the most popular programming language in the world, as it provides great flexibility and simplicity to write and build big applications on AI/ML, automation, web apps, desktop, etc.
One of the many reasons why Python is the top choice for programmers when developing their next application is its tons of features, with one such feature discussed in this article being its static type checking method.
At its core, Python lacks a static type-checking system, allowing programmers to initialize data without correctly specifying their type exclusively at first. Itβs a lifesaver for beginners unfamiliar with data types (though you should understand them), but often, instead of helping, this approach complicates maintaining functional code in large projects and can lead to runtime errors unless type annotations are specifically used within the code.
To assist developers, Microsoft launched Pyright, a free and open-source static type checker for Python written in TypeScript and running with Node. Itβs designed for performance and can effortlessly handle large Python source bases. The standout feature for me is its ability to run in βwatchβ mode, performing fast incremental updates when files are modified.
Features of Pyright
Pyright offers a bunch of features, and the best thing is that you can create a configuration file that provides granular control over settings for separate environments.
Currently, Pyright supports a lot of type-checking features, a few of which are listed below from the complete list:
- Syntax for variable annotations
- Type-hinting generics in standard collections
- Literal types
- Typed dictionaries
- Type parameter syntax
- Override decorator for static typing
- Marking deprecations
- TypedDict: read-only items
How to Install Pyright on Linux
The installation of Pyright can be done in two ways: first, if you are using VScode, you can simply install the Pylance extension, which offers Pyright type checking along with additional capabilities such as semantic token highlighting and symbol indexing.
However, if you are more of a command-line user and donβt rely on any IDEs, then you can easily install Pyright using PIP, Conda (maintained by the community), or NPM (officially maintained), and note that all of these methods require Node.
Install Pyright Using Pip or Condo
To install Pyright using Pip or Conda, ensure that the respective package manager is already installed, then run one of the following commands:
$ pip install pyright
#OR
$ conda install pyright
Once the installation is complete, the βpyright <options>
β command becomes accessible.
Install Pyright Using Node
Instead of using any other package manager like Pip or Conda, you can easily install Pyright using the officially supported NPM package manager.
$ sudo npm install -g pyright
After the installation, the βpyright <options>
β command becomes accessible.
How to Use Pyright on Linux
Once the installation is complete, Pyright will be accessible from your terminal. To verify, you can check its version by running the following command:
$ pyright --version
Output:

Now, to demonstrate its use case, Iβve used the following basic Python example, which greets a user and performs a simple calculation of the area of the room. After execution, the important thing to note is that the data type is not correctly specified in this code.
def greet(name: str) -> None:
print(f"Hello, {name}!")
def calculate_area(length: str, width: str) -> str:
area = length * width
return area
# Example usage
person_name: str = "Linux TLDR"
greet(person_name)
room_length: str = 5.0
room_width: str = 3.0
room_area: str = calculate_area(room_length, room_width)
print(f"The area of the room is {room_area} square units.")
When executing the above Python program, youβll find it executes successfully without displaying any errors.
$ python test.py
Output:

But when you pass this Python file to Pyright for type checking, youβll be surprised to see how many mistakes have been made in this program. See for yourself.
$ pyright test.py
Output:
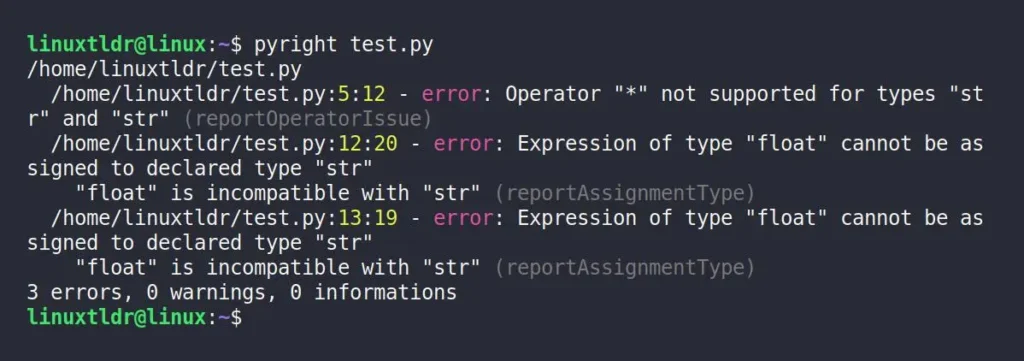
To prove that Pyright is not unreasonably showing errors, Iβve taken one step further and manually corrected the type mistake made in the Python program. The corrected version is shown below, with the syntax that has been corrected highlighted in green.
def greet(name: str) -> None:
print(f"Hello, {name}!")
def calculate_area(length: float, width: float) -> float:
area = length * width
return area
# Example usage
person_name: str = "Linux TLDR"
greet(person_name)
room_length: float = 5.0
room_width: float = 3.0
room_area: float = calculate_area(room_length, room_width)
print(f"The area of the room is {room_area} square units.")
When the corrected Python program is checked with Pyright, you will find that there are no errors, warnings, or information in the output.

It was a basic explanation of what Pyright can do, but you can accomplish more with it, and I highly recommend checking out its official documentation page to learn more about separate environment configuration and advanced usage.
Overall, itβs a great tool that you can use in your Python programming journey, with one annoying thing that I find, which is its dependency on NPM; apart from that, itβs a great tool that I recommend to everyone.
I hope you find this article useful; if you have any questions or queries related to the topic, please let me know in the comment section.
Till then, peace!
Join The Conversation
Users are always welcome to leave comments about the articles, whether they are questions, comments, constructive criticism, old information, or notices of typos. Please keep in mind that all comments are moderated according to our comment policy.