If you have been using Linux for a while, then you have definitely spotted this β#! /bin/bash
β line at the beginning of an shell script.
What is Shebang (#! /bin/bash) in Linux Shell Script
The β#! /bin/bash
β on Linux, famously known as shebang or hashbang, starts with a pound/hash (β#
β) sign together with an exclamation (β!
β) sign.
If you know quite a bit about shell scripting or the Linux command-line, you might know that in Linux, to specify a comment, you use the pound/hash (β#
β) sign.
The shebang is the only special exception that is not considered a comment when the hash/pound (β#
β) sign is specified at the beginning of a line.
What is the Purpose of Shebang in Linux Shell Script
To properly understand the purpose of shebang, you must be aware of the fact that Bash is not the only implementation of shell; shells like ZSH and Fish also exist.
Whenever you or a developer writes a shell script, they define the shebang at the beginning of the script to specify which shell interpreter will be used to run the given script.
So, if the first line of script is,
#!/bin/bash
Then the Bash interpreter will be used to run the given shell script.
If the following line is at the beginning of the shell script,
#!/bin/zsh
Then a ZSH interpreter will be used.
Also, it is allowed to have space between the shebang (β#!
β) and path of the shell (β/bin/bash
β).
#!/bin/bash #Without space will also work
#! /bin/bash #With space will also work
Why Shebang Is So Important in Linux Shell Script
Look if you have written a simple shell script like the one below.
echo "Sample Script"
Then it doesnβt much matter to include the whole shebang.
But the point is that most of the Linux interpreters, like Bash or ZSH, share common syntax and functions, but a few things might vary between these interpreters.
For example, if your shell script includes an array like the one below,
numbers=(one two three four)
echo ${numbers[1]}
Then, if you run the above script without specifying the shebang, you will get the following result from the Bash interpreter:
$ ./script.sh
two
The same script executed with the ZSH interpreter will return a different result.
$ ./script.sh
one
The difference occurred because Bash starts the array index at 0, and in ZSH, it starts at 1.
So, if the script is specifically intended to run in the Bash interpreter, then specify the shebang line at the beginning of the shell script.
#!/bin/bash
numbers=(one two three four)
echo ${numbers[1]}
If you run the above script, even if your systemβs default interpreter is ZSH, it will use the Bash interpreter to run this shell script.
But remember that mostly all Linux distributions use Bash as the default shell interpreter; if youβre using systems like Kali Linux or macOS, they provide ZSH as the default shell interpreter.
If you want your script to be specially run in the ZSH interpreter, you can specify it in the shebang line, but when the script is executed in a non-ZSH system, you will get the following error:
$ ./script.sh
bash: ./script.sh: /bin/zsh: bad interpreter: No such file or directory
Shebang Can Be Bypassed
When you run the shell script by specifying the relative or absolute path with the ZSH shebang, it will choose ZSH to run the specified script.
However, if you forcefully specify a different interpreter to run your shell script, then the shebang will be ignored, as shown.
$ cat script.sh
#!/bin/zsh
numbers=(one two three four)
echo ${numbers[1]}
$ bash script.sh
two
Output:
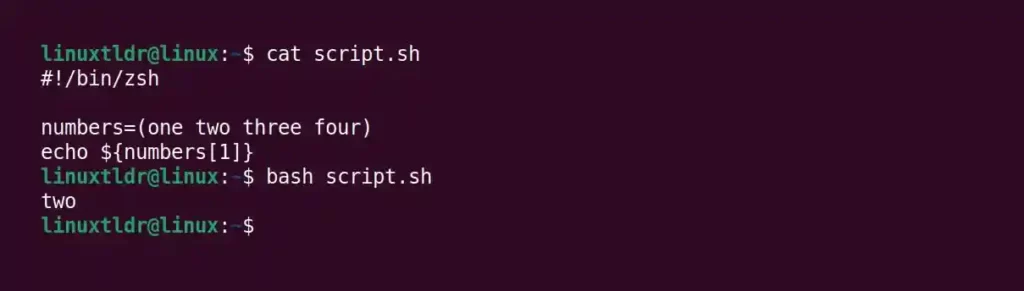
Shebang Is Not Only For Specifying Interpreters
Donβt underestimate the shebang is only used for specifying which interpreter must be used to execute a specified script.
The hash/pound with an exclamation (β#!
β) refers to the existing script, where β/bin/bash
β refers to the command.
#! /bin/bash
#BOTH ARE SAME#
bash file.txt
So, if you specify the cat command path instead of bash, it will print your whole script on the screen.
#!/bin/cat
numbers=(one two three four)
echo ${numbers[1]}
Output:
$ ./script.sh
#!/bin/cat
numbers=(one two three four)
echo ${numbers[1]}
It is possible to specify commands instead of the interpreter unless the block of lines is a valid argument for the given command.
So, that was the end of this article.
If you have any suggestions or queries regarding this topic, feel free to ask them in the comment section.
Join The Conversation
Users are always welcome to leave comments about the articles, whether they are questions, comments, constructive criticism, old information, or notices of typos. Please keep in mind that all comments are moderated according to our comment policy.