In this article, you will learn what the strip command is, its usecase, and how to use it to reduce the size of your compiled program.
Tutorial Details
Description | Stripping the Information from Compiled File |
Difficulty Level | Moderate |
Root or Sudo Privileges | No |
OS Compatibility | Ubuntu, Manjaro, Fedora, etc. |
Prerequisites | strip |
Internet Required | No |
Why Use the Strip Command?
Let’s consider you are a C programmer who writes a bunch of programs and uses the GCC compiler by executing the “gcc <program>
” command to compile your written program.
You might think that only your code is being compiled, but behind the scenes, the GCC compiler includes multiple pieces of information in your compiled file.
This includes the symbol information, relocation information, debug information, line number information, the comment section, file headers, etc.
This all-encompassing information, some of which you might never require, will increase the size of your compiled program, indirectly consuming more disk space.
What is the Strip Command in Linux?
The strip command is a GNU utility that is used to remove unnecessary information from your compiled file, reducing its size and making it more difficult to reverse engineer.
After removing the non-essential elements from your compiled file, your stripped file will consume less disk space and give you better performance.
Now, let’s see how you can use the strip command in Linux to remove the unnecessary elements from your compiled file.
How to Use the Strip Command in Linux
The following is a basic C program that will be used to demonstrate the use of the strip command.
#include <stdio.h>
int main() {
// printf() displays the string inside quotation
printf("Follow LinuxTLDR\n");
return 0;
}
You can copy and save the above code in the “program.c
” file in your existing directory and launch the terminal in the same directory.
Let’s compile the file and check its size after the compilation is finished.
$ gcc program.c -o compiled_file
$ ls -lh compiled_file
You can also run the program by executing the “./compiled_file
” command, as shown.

As you can see from the above picture, our program is compiled, and the current size of the compiled file is 16K.
Now let’s save the ELF information of this compiled file before stripping any information to use it as a comparison with the stripped file.
$ readelf -s compiled_file > compiled_file.txt
$ ls -lh compiled_file.txt
Output:

Here comes the fun part: let’s remove all the symbol and relocation information from the compiled file using the strip command with the “-s
” flag (harden the reverse engineering).
$ strip -s compiled_file
$ ls -lh compiled_file
Output:

Now, if you note the size of the stripped file, you will find the file size is reduced to more than 1K (from 16K to 15K), although it varies depending on the size and complexity of the program.
Now let’s save the ELF information of this stripped file by executing the following command:
$ readelf -s compiled_file > stripped_file.txt
$ ls -lh stripped_file.txt
Output:

Now let’s use the diff command to compare the previously saved ELF information (before stripping) and current ELF information (after stripping) to find out what type of information is removed.
$ diff compiled_file.txt stripped_file.txt
All the following information is removed from the “compiled_file
” program after stripping:
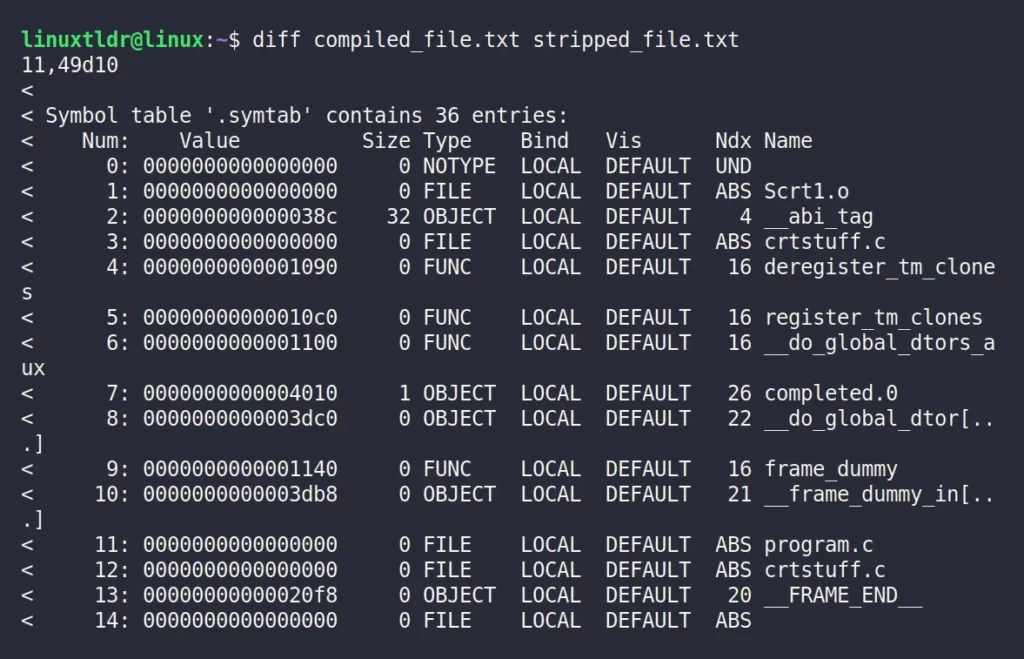
Now, if you are familiar with the “gcc -s
” flag, then you know that it also performs the same thing as the “strip -s
” flag does, so why use the strip in place of the gcc command?
The following are key reasons that favor using the strip command over the gcc command.
- Strip is used with compiled files while gcc is used as a compiler/linker and its “
-s
” flag strips the information while compiling. - While using strip you can use the “
-o
” flag to output the stripped file in a new file leaving the compiled file untouched. - Strip command provides you multiple flags to remove more specific information from your compiled file, for example “
--strip-debug
” flag will only remove the debug information from the compiled file.
Now let’s see a few more examples of strip command usage.
More Examples of Strip Command Usage
1. The following command will save the stripped file with a new name, leaving the compiled file untouched.
$ strip compiled_file -o stripped_file
2. The “--strip-debug
” flag will remove all the debug information from the compiled file.
$ strip --strip-debug compiled_file -o stripped_file
3. The “--only-keep-debug
” flag will remove everything except for debug information from the compiled file.
$ strip --only-keep-debug compiled_file -o stripped_file
4. The “--strip-dwo
” flag will remove all the DWO sections from the compiled file.
$ strip --strip-dwo compiled_file -o stripped_file
5. The “--strip-unneeded
” flag will remove all the symbols not needed by relocations from the compiled file.
$ strip --strip-unneeded compiled_file -o stripped_file
6. The “-M
” flag will remove all the redundant entries in note sections from the compiled file.
$ strip -M compiled_file -o stripped_file
To know more about the different flags and their information, check the strip command help section by executing the “strip -h
” command or the manual section by executing the “man strip
” command.
Let’s end this article here.
If you have any questions or queries related to this topic, then feel free to ask for help in the comment section.
Till then, bye bye.
Join The Conversation
Users are always welcome to leave comments about the articles, whether they are questions, comments, constructive criticism, old information, or notices of typos. Please keep in mind that all comments are moderated according to our comment policy.