Linux, a versatile and powerful operating system, offers an ideal environment for programming and, of course, for many other things. However, for newcomers transitioning from Windows to Linux, the installation of C/C++ tools can pose significant challenges.
Installing C/C++ development tools typically involves installing a compiler, such as GNU (GNU Compiler Collection), and related libraries such as make, libc-dev, dpkg-dev, etc.
The process may vary slightly depending on the Linux distribution you are using, but fear not, for this guide will teach you how to install the C/C++ compiler in Linux, complete with helpful examples.
Tutorial Details
Description | C/C++ Installation and Practical Usage |
Difficulty Level | Moderate |
Root or Sudo Privileges | Yes |
OS Compatibility | Ubuntu, Manjaro, Fedora, etc. |
Prerequisites | – |
Internet Required | No |
What is a Compiler?
In a nutshell, the compiler is specialized software that takes human-readable source code (written in C, C++, Java, etc.) and translates it into machine-readable code, also known as object code or machine code.
The CPU is capable of directly executing the translated code. Overall, the main purpose of a compiler is to help programmers write code in a more human-friendly manner while generating efficient and optimized machine code that can run on specific computer systems.
How to Install the C/C++ Compiler and Libraries in Linux
The following are some general steps to install C/C++ development tools and libraries on major Linux distributions:
Installing the C/C++ Compiler and Libraries on a Debian or Ubuntu System
For Debian/Ubuntu-based distributions, you can use the distributions default APT package manager to install the C/C++ compiler and related libraries:
$ sudo apt update && sudo apt install build-essential -y
Installing the C/C++ Compiler and Libraries on a Red Hat or Fedora System
For Red Hat/Fedora-based distributions, use the DNF or YUM package manager to install the C/C++ compiler and related libraries:
$ sudo dnf install gcc-c++ -y
#OR
$ sudo yum groupinstall "Development Tools" -y
Installing the C/C++ Compiler and Libraries in an Arch or Manjaro System
In Arch Linux and its derivatives (like Manjaro), you can install the C/C++ development tools and libraries by using the Pacman package manager:
$ sudo pacman -Sy base-devel
Verify the Installation
After the installation is successfully completed, verify that GCC (the C compiler) and G++ (the C++ compiler) are installed by running the following commands:
$ gcc --version
$ g++ --version
Output:
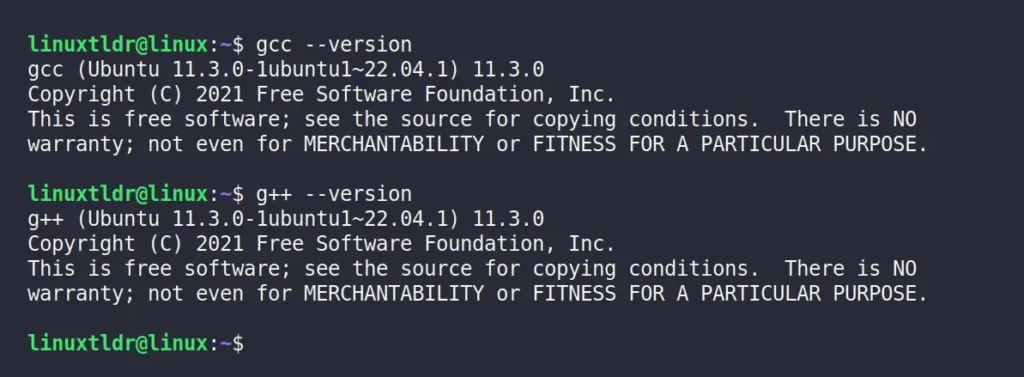
Testing C/C++ with Sample Programs
Running a C/C++ program requires the process of compiling and then running the compiled program. The following steps are involved and should be followed:
1. Write the Code: Create a new file with the “.c
” extension for C programs or the “.cpp
” extension for C++ programs to write your code (or paste the following respective code examples into this file).
2. Save the File: After finishing or pasting the sample code, save your file with an appropriate name, such as “hello.c
” for the C program or “hello.cpp
” for the C++ program.
3. Open the Terminal: Open a terminal in the same directory where your C/C++ program files are located.
4. Compile the Code: Use one of the following commands based on the requirement to compile a C/C++ program:
$ gcc -o hello hello.c #For C programs
$ g++ -o hello hello.cpp #For C++ programs
The “-o
” flag is used to specify the output filename of the compiled executable. In this case, we name it “hello
“, but you can choose another name if you prefer.
5. Run the Program: Lastly, execute the compiled file using the output filename followed by “./
” syntax in Linux.
$ ./hello
After executing the above command, you will see the output of the program displayed on the terminal.
C/C++ Sample Programs
The following are some simple examples of C/C++ programs:
#C Example: Hello World
#include <stdio.h>
int main() {
printf("Hello, LinuxTLDR!\n");
return 0;
}
Output:

#C++ Example: Hello World
#include <iostream>
int main() {
std::cout << "Hello, LinuxTLDR!" << std::endl;
return 0;
}
Output:

#C Example: Addition of Two Numbers
#include <stdio.h>
int main() {
int num1, num2, sum;
printf("Enter two numbers: ");
scanf("%d %d", &num1, &num2);
sum = num1 + num2;
printf("Sum: %d\n", sum);
return 0;
}
Output:

#C++ Example: Addition of Two Numbers
#include <iostream>
int main() {
int num1, num2, sum;
std::cout << "Enter two numbers: ";
std::cin >> num1 >> num2;
sum = num1 + num2;
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Output:

Important GCC/G++ Options
The compilation of C/C++ programs can be done by following the standard method mentioned earlier.
But using the extra options while compiling will allow you to control the compilation process, enable specific features, and optimize the resulting binary.
The following are some important GCC (for the C Program) options:
Options | Usage |
---|---|
-o <output-file> | Specify the output filename for the generated executable or object file. |
-Wall | Enable all compiler warnings and messages to catch potential issues and coding problems. |
-Werror | Treat all warnings as errors, and stop the compilation if any warnings are present. |
-std=<standard> | Specify the C++ language standard to use, such as “-std=c89 “, “-std=c99 “, “-std=c11 “, etc. |
-O<level> | Enable optimization. Replace “<level> ” with a number from 1 to 3 or s for size optimization. |
-g | Include debugging information in the generated executable or object file for use with debuggers like GDB. |
-I<include-path> | Add directory to search for header files. |
-L<library-path> | Add directory to search for libraries during linking. |
-D<macro> | Define a preprocessor macro during compilation. |
-c | Compile the source files and generate executable or object files without linking. |
The following are some important G++ (for the C++ Program) options:
Options | Usage |
---|---|
-o, -Wall, -Werror, -O, -g, -I, -L, -D, -c | The referenced GCC (for C Program) options will also work for G++ (for C++ Program). |
-Wextra | Enable extra warning messages in addition to those enabled by “-Wall “. |
-std | Specify the C++ language standard to use, such as “-std=c++98 “, “-std=c++11 “, “-std=c++14 “, “-std=c++17 “, “-std=c++20 “, etc. |
-l<library> | Link against the specified library. For example, “-lm ” will link to the math library. |
-fno-exceptions | Disable C++ exception handling. |
-fno-rtti | Disable C++ Run-Time Type Information (RTTI). |
Final Word
I hope this article will be useful for you. If something is missing or you have any recommendations for modification, then feel free to tell us in the comment section.
Till then, peace!
Join The Conversation
Users are always welcome to leave comments about the articles, whether they are questions, comments, constructive criticism, old information, or notices of typos. Please keep in mind that all comments are moderated according to our comment policy.