In this article, you will learn what the difference is between variables and parameters, what special parameters are, and a list of predefined special parameters in Bash.
What are βSpecial Parametersβ in Bash?
Before you can understand what special parameters are, you must first understand what parameters are: Any entity that stores a value (also known as a variable) is the parameter.
Yes, itβs that short in definition; now, special parameters are a few predefined parameters given by your shell that hold read-only information related to your existing shell session.
This parameter can be used in your terminal or shell script to control how the script runs by figuring out the userβs shell session or getting the arguments given to the script by user.
List of Bash Special Parameters
The following is the list of known predefined special parameters served by your shell (basically Bash).
Special Parameters | Description |
---|---|
$$ | Return the PID of an existing Bash shell. |
$! | Return the PID of the latest executed command. |
$# | Count the number of positional arguments specified to the script in decimal. |
$0 | Print the script name. |
$? | Display the exit status of the last executed command. |
$_ | Return the last argument specified to the script. |
$i | The βi β refers to the position of the argument; for example, the β$1 β parameter returns the first argument, and the β$2 β parameter returns the second argument specified to the script. |
$@ | Return each of the arguments passed to script separately. |
$* | Identical to the β$@ β parameter, except that when specified inside quotes, it treats all arguments as a single argument. |
$- | Return the current flag assigned to your Bash shell. |
Prerequisite
Few of the special parameters can be shown in the terminal, but some must be shown in the shell script for you to fully understand how to use them.
For that reason, check out our article on how to run shell scripts in Linux to create scripts when they are required.
Displaying the PID of an Existing Bash Shell
The β$$
β parameter holds the PID information of your existing bash shell; for example, when you echo the value of this parameter, you will find the PID of your existing bash shell.
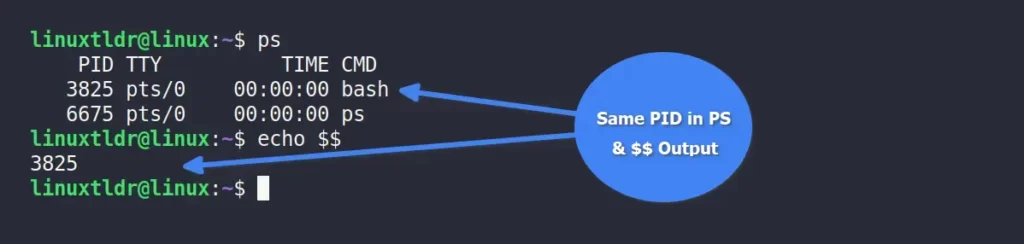
Note that when the β$$
β parameter is used inside the script, it will return the PID of the script (executed in a subshell) itself.
Displaying the PID of the Last Executed Command
Unlike the β$$
β parameter, the β$!
β parameter returns the PID of the latest executed command in your current shell session.
The following command will return the PID of the sleep command in the output.

Counting the Number of Arguments Assigned to Shell Script
The β$#
β parameter is mostly used inside the shell script to count the number of arguments assigned to the referenced shell script at the time of execution.
The following is the simple script that echoes the β$#
β parameter when executed.

If the user specified two arguments while running the above script, the β$#
β parameter will return 2 in the output.

If the user specified five arguments while running this script, the β$#
β parameter will return 5 in the output.

Printing the Referenced Script Name
The β$0
β parameter is used to print the name of the shell or referenced shell script. As a result, you can use this to print the name of the shell script.
The following is the simple shell script echoing the β$0
β parameter, when script successfully executes.

When the above script is executed, it will return the name of the script itself.

Note that when you use this parameter in the terminal, it will return Bash, as this is the first process before your command is used to run.

Displaying the Exit Status of the Last Executed Command
The β$?
β parameter is used to get the exit status of the most recently executed command in the current shell session; we have already written a detailed guide on this topic that you can check.
But for your reference, check the following list:
0
: Successful execution.1
: General error.2
: Shell built-in errors.127
: Command not found.
In the following example, one is the right command that was executed successfully, returning β0
β as the exit status code, and another is the wrong command that failed to execute and returned the β127
β exit status code.
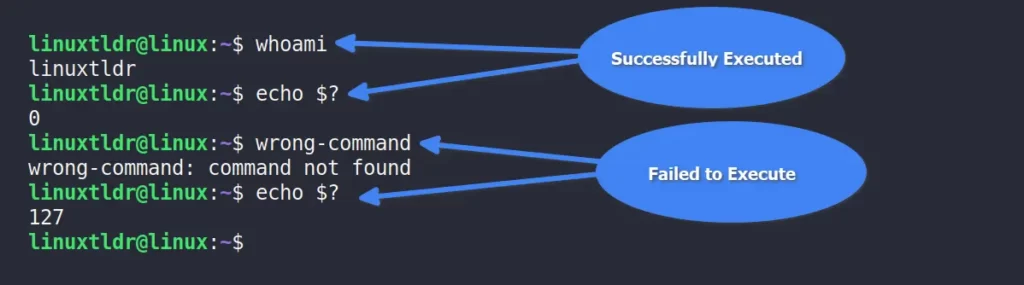
Note that this parameter is a crucial part of the shell scripting process that is used to control the flow of script in different situations based on the last exit status code.
Displaying the Last Argument Provided to the Previous Command
The β$_
β parameter will display the last argument (or positional parameter) assigned to the previous command, whether in a terminal or shell script.
In the following example, the β$_
β parameter will return the last argument of the previous ps command, which is the β-r
β flag.
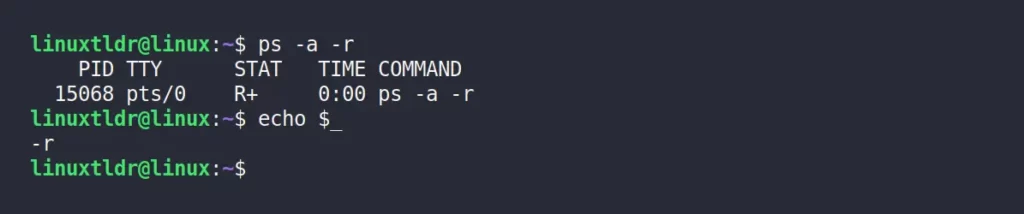
Displaying the Specific Argument Based on Position
The β$i
β parameter, where βi
β is referred to as the argument position in β$1
β or β$2
β assigned to shell script at the time of execution.
In the following example, we used the β$1
β parameter, which returns the first argument passed to the shell script.
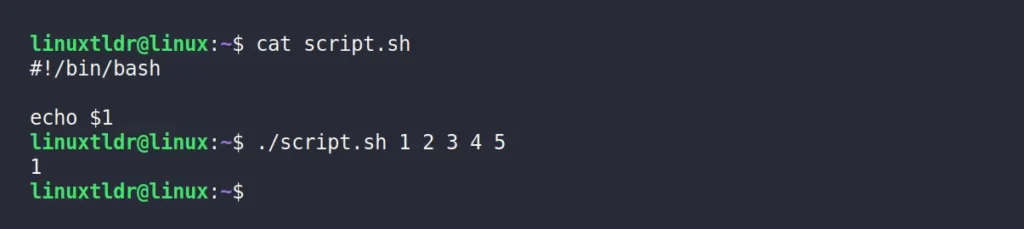
In the next example, we used the β$2
β parameter, which returns the second argument that was given to the shell script.
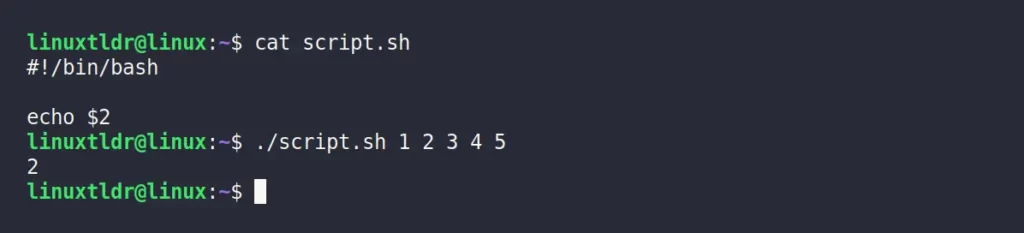
Displaying All of the Shell Script Arguments using $@ Parameter
The β$@
β parameter will return all the specified arguments assigned to the referenced shell script, separately.
In the next example, we used the β$@
β parameter, which tells the referenced shell script to return all the arguments that were given to it.
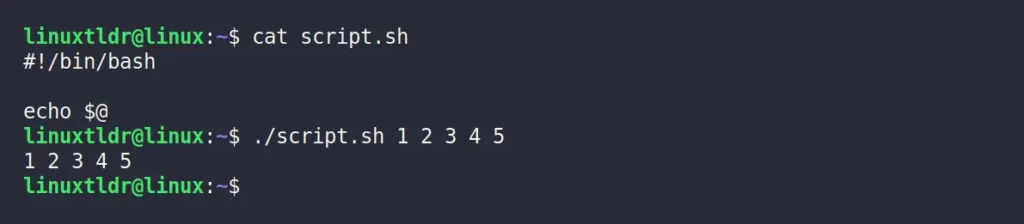
Displaying All of the Shell Script Arguments using $* Parameter
The β$*
β parameter is identical to the β$@
β parameter when specified without quotes, as shown.
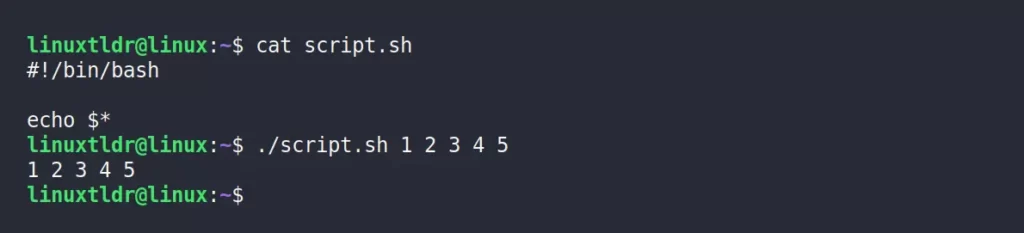
Difference Between the $* and $@ Parameters
The nature of this parameter depends on whether it is specified with or without quotes.
Case 1: No quotes around $*
and $@
parameters:
In this case,
The [./script.sh arg1 arg2 arg3
] with [$*
] gets [arg1
, arg2
, arg3
] as separate arguments.
The [./script.sh arg1 arg2 arg3
] with [$@
] gets [arg1
, arg2
, arg3
] as separate arguments.
In the following script, the β$*
β and β$@
β parameters are placed without quotes using a βfor loop
β in bash:
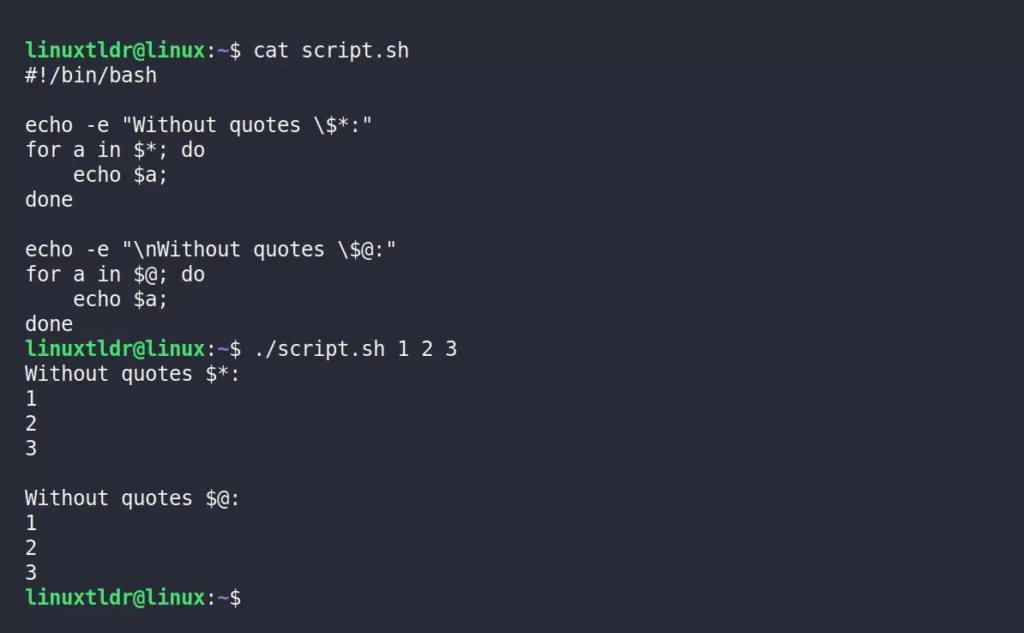
As you can see without quotes, both parameters treat the user assigned arguments separately.
Case 2: With quotes around the β$*
β and β$@
β parameters:
The [./script.sh arg1 arg2 arg3
] with [β$*
β] gets [arg1 arg2 arg3
] as a single argument.
The [./script.sh arg1 arg2 arg3
] with [β$@
β] gets [arg1
, arg2
, arg3
] as separate arguments.
In the following script, the β$*
β and β$@
β parameters are placed inside quotes using a for loop
in bash:
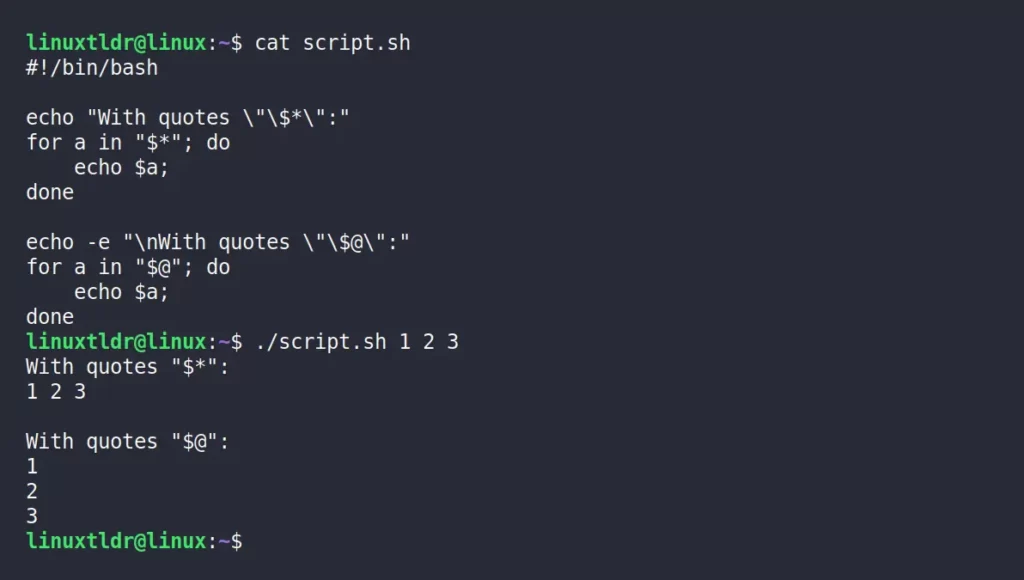
In this case, when both the parameters are assigned inside quotes, the β$*
β treats each assigned argument as a single argument, and the β$@
β treats each as a separate argument.
Displaying the Current Flag Set to Your Bash Shell
The β$-
β parameter is used to retrieve the current flags assigned to your Bash shell, whether they were set using the set built-in command or by the bash shell itself.

The βhimBHs
β flag is assigned to my bash shell.
Where:
H
β histexpandm
β monitorh
β hashallB
β braceexpandi
β interactive
Wrap Up
The special parameter is mostly used in shell scripts to perform various tasks like checking the exit status of a recent command or fetching the user assigned arguments.
If any parameters were missed in this article that should have been mentioned, then do let us know in the comment section.
The βList of Bash Special Parametersβ table at the start of the article has the wrong command for listing the exit status of a command. It shows $! when it should be $?. The explanation for the command later in the article shows the correct command.
Thanks for noticing that, Gary. Article has been updated now.