Polyglot programming is uncommon but can be a lifesaver in specific situations. For example, we recently wrote an article about executing Python scripts within a PHP/HTML file, which can be valuable for certain programmers.
This approach proves useful in scenarios such as running system diagnostic scripts or gathering information based on specific triggers. To learn more, you can refer to the article, but today our focus will be on executing JavaScript within Python.
Can you Execute JavaScript Within Python?
Your first question might be: Is it possible to execute JavaScript in Python? The answer is yes. However, those unfamiliar with this concept and reading this article solely for additional knowledge might wonder why someone would want to run JavaScript within Python.
There are several scenarios where one might want to do that, such as integrating existing JavaScript code, web scraping and automation, cross-language testing, data manipulation, and many other situations.
Now, this task can also be accomplished internally through Python code itself, but it depends on the situation. For example, if you have existing JavaScript code that is compatible with your Python environment without requiring significant resources, why not opt for it instead of writing the entire code from scratch in Python?
There are many scenarios like this that I could talk about, but let’s not waste our time on that and instead focus on how one can run JavaScript in Python.
How to Run Javascript in Python
JavaScript can be executed in Python through various methods; for example, specific Python libraries such as PythonMonkey, PyExecJS (deprecated), and js2py can help you accomplish this.
PythonMonkey supports and runs JavaScript code exclusively on the Mozilla SpiderMonkey engine. PyExecJS is popular, offering an API to run JavaScript code from Python using various engines like Node.js, JavaScriptCore, and Google V8.
Lastly, js2py is written purely in Python and doesn’t depend on any JavaScript engine. Instead, it translates JavaScript code (which supports ECMA-6) and interprets it in Python.
First, let’s check the prerequisites for running JavaScript within Python, followed by practical demonstrations.
Prerequisites
Before we begin, ensure that Python with pip (also including Node.js would be better) is installed and properly configured on the system where you intend to carry out the practical tasks described in this article.
Secondly, ensure you have an installed GUI-based text editor like VSCode or Geany for a better experience. In conclusion, let’s start with our first method.
Method 1: Run Javascript in Python using PythonMonkey
PythonMonkey is still in active development, yet it provides a better way of running JavaScript in Python by embedding the Mozilla SpiderMonkey JavaScript engine into the Python VM and using the Python engine to provide the JS host environment.
To begin, install PythonMonkey by executing the following command:
$ pip install pythonmonkey
After installation is complete, you can effortlessly import the library into your Python project and utilize the “eval()
” function for evaluating JavaScript code. For instance, the following program will execute JavaScript code as defined in “console.log()
“.
from pythonmonkey import eval as js_eval
js_eval("console.log")('Hello from Linux TLDR')
Output:
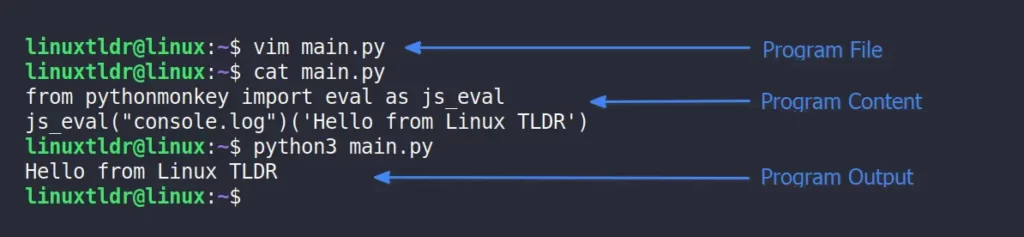
In the above example, you learned to run JavaScript code internally within the same Python program. If you have an external JavaScript file, you can use the “require()
” function from PythonMonkey to load a JavaScript module into Python and call functions defined in the external JavaScript.
For example, we have two files, “math-script.js
” and “main.py
“, where the JavaScript function defined in the “math-script.js
” file will be called in the “main.py
” Python file.
Content of the “main.py“.
from pythonmonkey import require as js_require
js_lib = js_require("./math-script")
print(js_lib.add_func(1,6)) # 7
print(js_lib.sub_func(1,6)) # -5
Content of the “math-script.js“.
// Javascript function for addition and substraction
function add_func(a,b) {
return a + b;
}
function sub_func(a,b) {
return a - b;
}
// Here, define the exports object just like in Node.js or NPM modules.
module.exports = {
add_func,
sub_func,
}
Now, when “main.py
” executes, the JavaScript content from “math-script.js
” will be used to evaluate the assigned values, and the resulting output will be printed.
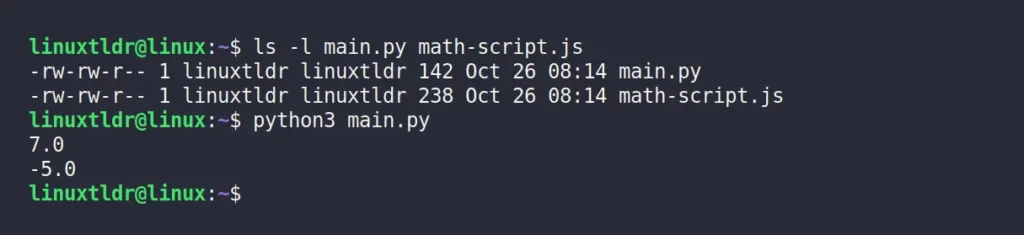
Here, I must stop, as there are endless examples that can be shown. If you’re wondering whether complex JavaScript functionality like promises or fetching APIs can be performed, the answer is yes.
So, if you loved these libraries and want to continue your journey further, I suggest you check out a few basic to advanced examples from here.
Method 2: Run Javascript in Python using PyExecJS
PyExecJS was once quite popular, and the reason I mention this is that it’s now deprecated. However, I still use it in certain situations, and, of course, it can still handle basic tasks.
So, to begin, install the library using the Python package manager.
$ pip install PyExecJS
Next, you can paste the provided Python program, where a bunch of JavaScript code is defined within the “js_code
” variable and executed using the “compile()
” and “eval()
” functions from the imported “execjs
” library.
from execjs import compile as js_runner
# JavaScript code
js_code = """
function add_func(a, b) {
return a + b;
}
var result = add_func(2, 3);
"""
# Use compile function from execjs to execute the JavaScript code
exec_js = js_runner(js_code)
# Get the result of the JavaScript code
result = exec_js.eval("result")
# Print the result
print(result)
Now, since this library has reached its end of life (EOL), I won’t go further into a detailed explanation. However, if you’re interested in learning some hands-on techniques, feel free to check out its GitHub page.
Method 3: Run Javascript in Python using js2py
Js2py is another quite popular library for running JavaScript in Python, as it’s entirely written in Python. Like previous libraries, it doesn’t require any JavaScript runtime; instead, Python and pip are sufficient to kickstart your journey.
To begin, type the following command in your terminal to install the js2py library:
$ pip install js2py
Now, we will explore a basic example of using this library in which a simple greeting message assigned to the “js_code
” variable will be printed.
import js2py
js_code = """
console.log("Hello from Linux TLDR");
"""
context = js2py.EvalJs()
context.execute(js_code)
The “js2py.EvalJs()
” function establishes a JavaScript execution context, and “context.execute(js_code)
” runs the JavaScript code within that context, producing the output:

Now, you can use an external JavaScript file in your Python program, but I personally do not recommend it because it’s written entirely in Python, limiting the scope to a restricted area due to the unavailability of many JavaScript functionalities.
However, you can explore further by visiting this library’s GitHub page.
Final Word
Now, you might be wondering which of the three libraries (excluding one that is deprecated) to choose for performing the same task. My answer would be: if you are unfamiliar and new to the topic, go for PythonMonkey without a doubt.
It’s simple yet can be used to perform basic to advanced JavaScript operations easily; the tutorial is readily available on the internet, and I personally use it, so feel free to ask for help in the comment section.
Till then, peace!
If you need to call JavaScript functions Python, use PythonMonkey! It’s the fastest and most standardized python library for python & javascript interop.
Thanks for writing this article Linux TLDR team!
╰(*°▽°*)╯
PythonMonkey is a really cool concept, I like being able to pass variables into JS without using CLI variables or args, as I would if I was calling the JS code through the shell with something like: os.system(‘node script.js’). Is there a way to pass variables back from JS into the calling Python script?
I haven’t found any method to access JS values in Python within PythonMonkey. Whenever I need to accomplish this task, I go with PyExecJS. If I come across a solution, I’ll edit this comment with the solution.
Maybe I’m misunderstanding, but I think they technically do that in the js_require example!
“`print(js_lib.add_func(1,6))“`
is passing the result of `js_lib.add_func(1,6)` back to Python. I imagine you could do more complex stuff with a function that returns multiple values, like:
“`from pythonmonkey import require as js_require
js_lib = js_require(“./generateRandomNumbers”)
fiveRandomNumbers = js_lib.generate(5)) # [5, 27, 13, -2, 0]“`