In this article, you will learn how to run a Python script on a PHP (or even a plain HTML) file using two different methods, along with practical examples.
I assume you have already installed Python with Pip and set up the web development environment on your Linux system, with the web root directory located at β/var/www/html
β, and that you have the necessary root or sudo permissions.
Before we continue, itβs important to note that running Python scripts with GUI operations like Matplotlib or Tkinter requires external tools, while CLI programs like NumPy or Pandas can run using built-in functions.
Keeping all of this in mind, letβs learn how to run a Python script in a PHP or HTML file on your Linux system.
Practice Python File for Demonstration
In this example, Iβll use two different Python scripts: one is a basic script that outputs βHello from Linux TLDR
β, and the other is a slightly more complex file that utilizes NumPy for complex mathematical operations.
Python Script 1: Displaying a simple βHello from Linux TLDR
β output
def main():
print("Hello from Linux TLDR")
if __name__ == "__main__":
main()
Python Script 2: Performing complex mathematical operations using the NumPy library
pip install numpy
β command to initiate the installation.import numpy as np
# Create a NumPy array from a list
my_list = [1, 2, 3, 4, 5]
numpy_array = np.array(my_list)
# Print the NumPy array
print("NumPy Array:")
print(numpy_array)
# Basic operations with NumPy arrays
print("\nBasic Operations:")
print("Sum:", np.sum(numpy_array))
print("Mean:", np.mean(numpy_array))
print("Standard Deviation:", np.std(numpy_array))
# Create a 2D NumPy array
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Print the 2D NumPy array
print("\n2D NumPy Array:")
print(matrix)
# Transpose the 2D NumPy array
transposed_matrix = np.transpose(matrix)
# Print the transposed 2D NumPy array
print("\nTransposed 2D NumPy Array:")
print(transposed_matrix)
I suggest you use the above Python script for demonstration purposes, copy its content, and save it in the β/var/www/html
β directory with the file names βpy_script1.py
β and βpy_script2.py
β for the first and second Python script, respectively.
Here is how your directory structure would look with the output of both Python scripts.
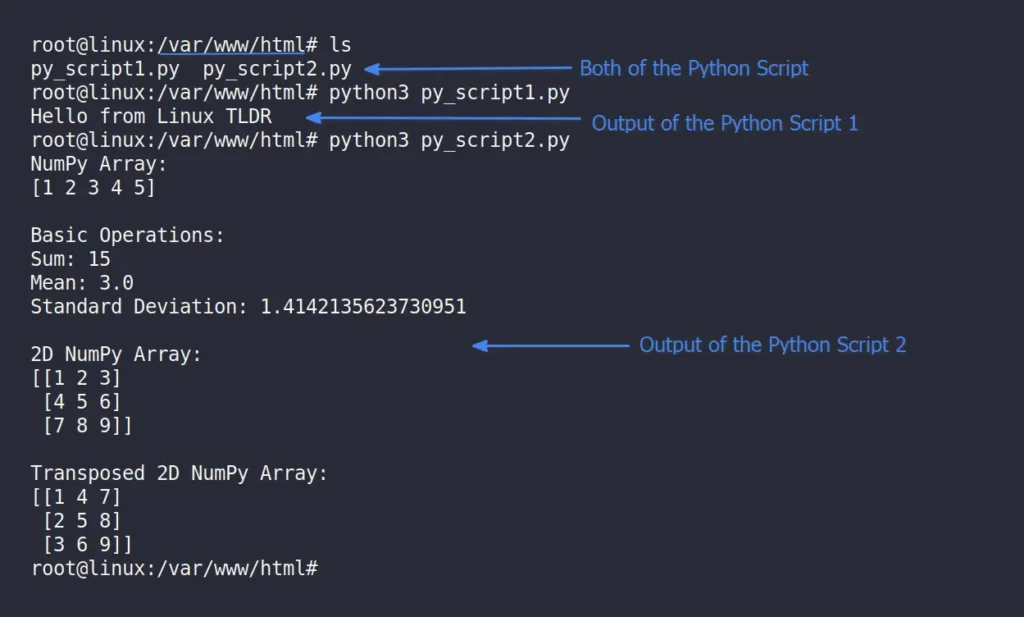
So, letβs start with the first method to run a Python script on PHP without using any external tools.
Method 1: Run Python Scripts on PHP File Without External Tools
Begin by creating a PHP file in the β/var/www/html
β web root directory with the name βindex.php
β and pasting the following PHP code into it:
<?php
$pythonScript = "py_script1.py";
exec("python3 $pythonScript", $output, $returnCode);
if ($returnCode === 0) {
echo implode("\n", $output);
} else {
echo "Error running Python script. Return code: $returnCode";
}
?>
In the above program, we first execute the βpy_script1.py
β file, and do not forget to replace the βpython3
β command with the one you use to initiate the Python interpreter on your Linux system.
When accessing the βindex.php
β page from your browser via βlocalhost/index.php
β or your IP address, you will get the following Python script output:
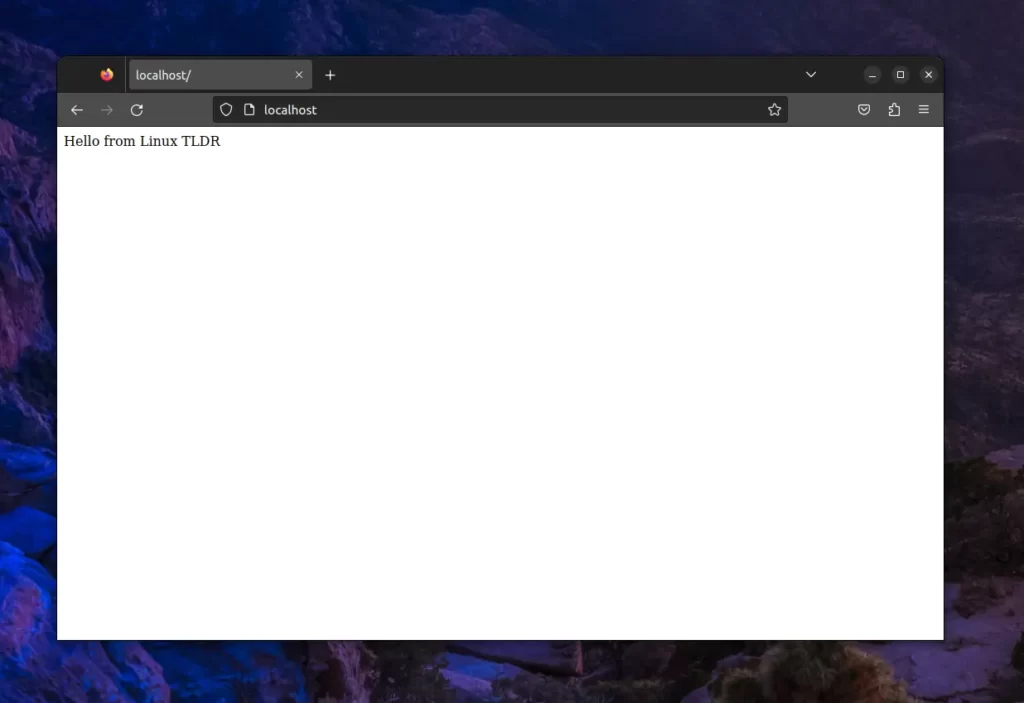
Likewise, you can replace βpy_script1.py
β with βpy_script2.py
β in the previously mentioned PHP script to execute the Python script containing NumPy code and then refresh the page.
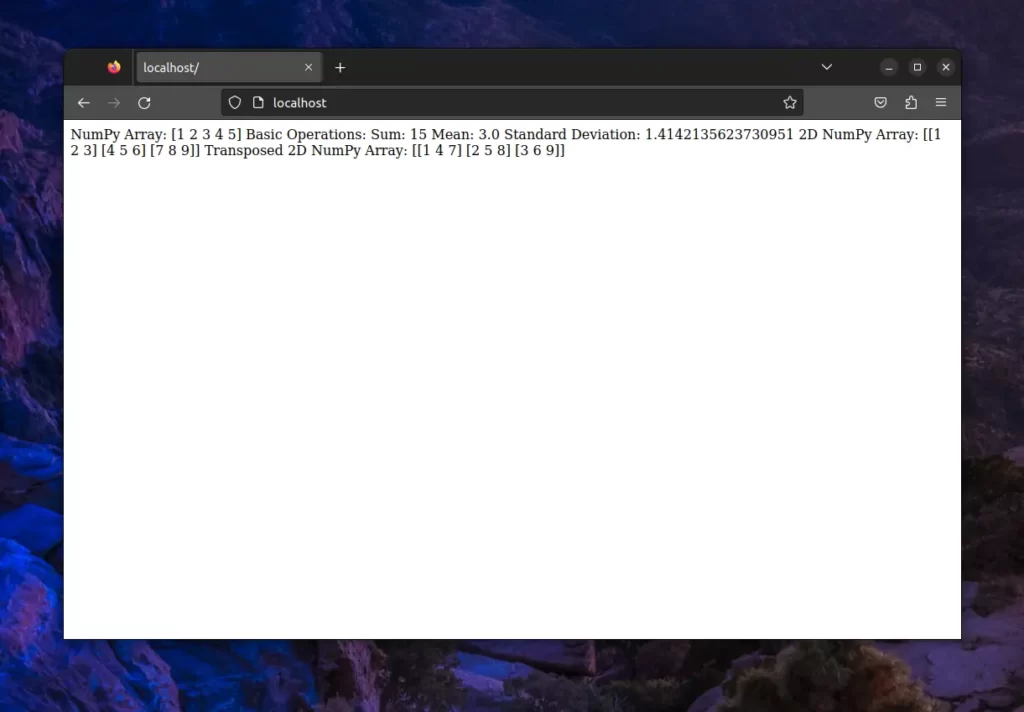
The result lacks formatting due to encapsulation before printing, but it functions as intended. Unfortunately, this method doesnβt support running Python scripts with GUI operations.
Now, if we discuss the code used in the Python script, it includes some PHP variables, the βexec()
β function, and an if-else statement.
Most things are self-explanatory; the most important one is the βexec()
β function, which is used to execute a command via the shell and returns the last line of the commandβs output.
It enables the execution of external programs or shell commands, commonly used for running system commands, executing scripts, or performing similar tasks on the server where PHP is operating.
But remember to use it cautiously, as this function will execute the script on your Linux system, granting all permissions and enabling system modifications.
Method 2: Run Python Script on an HTML File Using PyScript
This method can help you achieve the same task in your HTML file using the PyScript framework, which allows any HTML file to run Python scripts.
With PyScript in your HTML file, you can also run a Python script performing GUI operations like Matplotlib, Altair, Folium, etc., and further, you can write Python code in your HTML file.
The only complaint I have with this tool is that it launches an integrated terminal within the web page, displays the output of the Python script in the terminal, and downloads the necessary components each time you refresh the page.
But for now, letβs set this aside and explore how you can use PyScript to execute Python scripts or code in your HTML file.
I assume you have at least a little knowledge of HTML, so create a basic HTML template and add the following PyScript CDN in the head tag.
<link rel="stylesheet" href="https://pyscript.net/latest/pyscript.css" />
<script defer src="https://pyscript.net/latest/pyscript.js"></script>
Next, each library that your Python script or code requires should be included under the β<py-config>
β tag within the βpackages
β variable in an array.
Your Python code should be written under the β<py-script>
β tag. If you are importing an external Python script from your system, use the βsrc
β attribute to specify the path of the Python script.
In my case, I will directly run the βpy_script2.py
β file, importing the NumPy library, so my HTML file will look like the one below.
<html>
<head>
<!-- PyScript CDN -->
<link rel="stylesheet" href="https://pyscript.net/latest/pyscript.css" />
<script defer src="https://pyscript.net/latest/pyscript.js"></script>
</head>
<body>
<!-- Greeting from Linux TLDR -->
<div style="text-align: center">
<h1>Running Python Script/Code in your HTML file</h1>
<p>Thanks for following <a href="https://linuxtldr.com">Linux TLDR</a></p>
<div>
<!-- Importing supporting libraries for require Python Script/Code -->
<py-config>
packages = ["numpy"]
</py-config>
<!-- Your Python Script/Code -->
<py-script src="py_script2.py">
</py-script>
</body>
</html>
If you are following along with me, you can copy the HTML code above, save it in an βindex.html
β file within your β/var/www/html
β directory, and then navigate to βlocalhost/index.html
β in your web browser to access it.
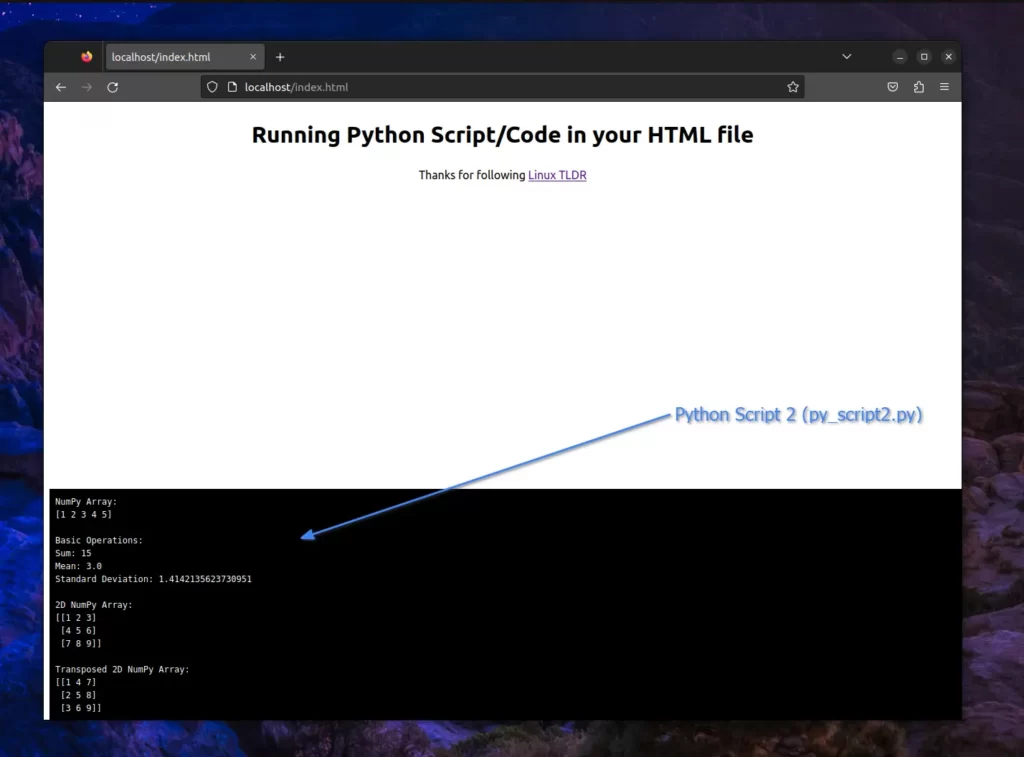
In this way, you can execute any Python script or write Python code in your HTML file using the PyScript framework. The only limitation is that you cannot interact with Python as you would with PHP, and the output is displayed in the terminal.
If you are creating a βTry it Outβ editor or showcasing the functionality of any Python script on your web page, this could be a great solution.
If youβd like to dig deeper into this framework, be sure to visit its documentation page or head directly to the examples page if you have a basic understanding of HTML and JavaScript.
Final Word
Thereβs nothing left to say since most questions are already answered in the article, but if you have any, feel free to let us know in the comment section.
Till then, peace!
Bro, you saved me a lot of time! BTW, PyScript is inconvenient at the beginning but works great after reading a little documentation.
I loved knowing this article helped you!