How do I write and run a shell script on Linux? Is there a way to run a shell script without executable permission, or can we run a shell script directly from a URL? Don’t worry; you are about to learn all those things in this article.
So, let’s begin by writing a simple shell script. For this, you can use your preferred text editor; I usually prefer Nano or Vim, but you can also use a GUI editor like Norka or VSCode.
Once you’ve decided, create a simple file, copy-paste the following line that basically prints a simple message on the screen using the echo command, and once done pasting, save your file as “script.sh
” (the “.sh
” extension indicates it’s a shell script file).
echo "Hello, It's Linux TLDR"
Now that our shell script file is ready, there are multiple ways to execute the shell script in Linux, followed by:
- Running the shell script using the “
bash
” command and specifying the script filename as an argument. - Running the shell script by specifying its absolute or relative path.
- Running the shell script directly from a URL.
- Running the shell script with a different shell interpreter.
- Running the shell script through the Desktop Environment File Manager (e.g., Nautilus).
So, let’s start by running the shell script using the “bash
” command.
Method 1: Running the Shell Script Using the Bash Command
Bash is a superset of the traditional shell program, which comes with more features out of the box. You can use this program to run your shell script, and the best part is that you do not need executable permission for your script file.
So, to run a shell script, use the bash command, followed by the script’s filename.
$ bash script.sh
Output:

Prior to moving forward, I want to draw your attention to one thing: the shell script is like a program file, and the shell interpreter, such as bash (the one we have been using until now), can be regarded as another interpreter, such as for Python or Javascript.
So, whenever you write a shell script, ensure you use the correct syntax, keywords, or commands valid for that particular shell interpreter. We’ll learn about the differences between different shell interpreters later in this article; for now, let’s explore how you can run a shell script using the path.
The source command in Linux can be used to read and execute the commands from the shell script file (in order) as its argument in the current shell.
$ source script.sh
Method 2: Running the Shell Script by Specifying its Path
In the previous method, you learned how to run a shell script using the bash command, but you can easily run your shell script from its relative or absolute path without the bash command.
The limitation of this method is that you need executable permission to run the shell script; if you attempt to execute it without that, it will throw the following “Permission denied
” error:

To avoid this error, assign executable permission to your shell script using the chmod command.
$ chmod +x script.sh
$ ls -l script.sh
Output:

Once you’ve assigned the executable permission, you can easily execute the shell script by specifying its relative path with “.
” (dot) as a prefix.
$ ./script.sh
Output:

If you’re wondering why “.
” (dot) is used as a prefix, it’s because the shell script we’re executing is in the current directory, and that dot represents the current working directory. However, if you execute the same shell script by its absolute path, that dot is not necessary, and you can run your shell script without it.
$ /home/linuxtldr/script.sh
Output:

Would you like to execute your shell scripts from anywhere without using the bash command or specifying their path? Simply create a directory, place all your shell scripts in that directory, and add that directory to the $PATH environment variable.
The following is a simple syntax for adding the “/path/to/your/bash/directory
” path to your $PATH environment. You can execute it directly in your terminal, but note that the changes will only be valid for the current shell session. To make the changes persistent, you should add this line at the end of your shell configuration file.
$ export PATH="/path/to/your/bash/directory:$PATH"
Once you’re done adding, you can access the shell script in that directory from anywhere.
Method 3: Running the Shell Script Directly from a URL
So far, all the methods we’ve seen involve running a shell script from the local machine. However, if you find a shell script on the internet, such as one hosted on GitHub Gist, you can either download and execute it using any of our previously mentioned methods, or you can directly execute it from the URL using the “curl
” or “wget
” command.
For example, I copied the following shell script’s URL in raw format from GitHub Gist:
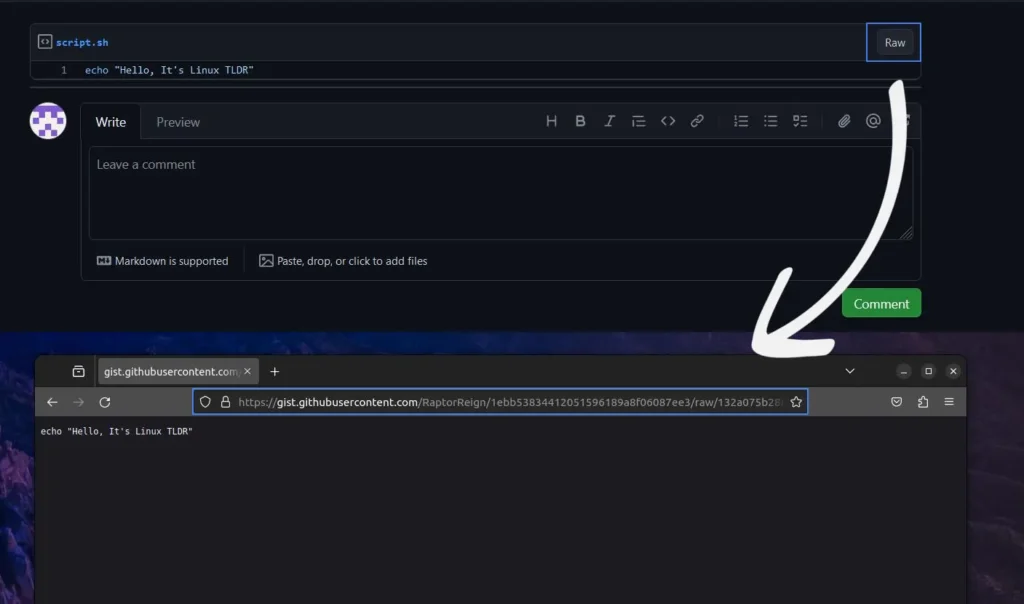
To execute this shell script directly from the URL, I can use either the following curl or wget command:
$ curl -s https://example.com/linuxtldr/script.sh | bash
#OR
$ wget -qO - https://example.com/linuxtldr/script.sh | bash
Output:

For curious minds wondering how this happened, we basically first download the script into temporary memory, then redirect it to the bash command with a pipe. With this method, you can avoid manual work and perform shell script execution tasks in one go.
Method 4: Running Shell Script With a Different Shell Interpreter
Thus far, we’ve used the bash interpreter to run our shell script; however, as mentioned at the beginning of this article, bash isn’t the sole interpreter available. Linux distributions such as Manjaro and Kali Linux have begun shipping Zsh as the default login shell.
In addition to Zsh, there are other shell interpreters such as Fish, C shell, Korn shell, etc., each with its own advantages and disadvantages. Today, most Linux distributions come with Bash, which is why we prefer it over others. However, if you want to force users to run your shell script from a different shell interpreter, you can use Shebang.
The Shebang is not a program or tool; it’s just a method for specifying the full path of the shell interpreter after “#!
” syntax. For example, to force a user to run your shell script in bash on Manjaro or Kali Linux, you can add “#! /bin/bash
” at the beginning of your shell script.
To demonstrate, I’m using Kali Linux with Zsh as the login shell, so in the following example, I’ll first check my current login shell, then show you the content of the shell script where the Shebang is specified, later executing it using the relative path.
# Checking the existing login shell.
% echo $0
# Checking the content of shell script.
% cat script.sh
# Executing the shell script from a bash interpreter.
% ./script.sh
Output:
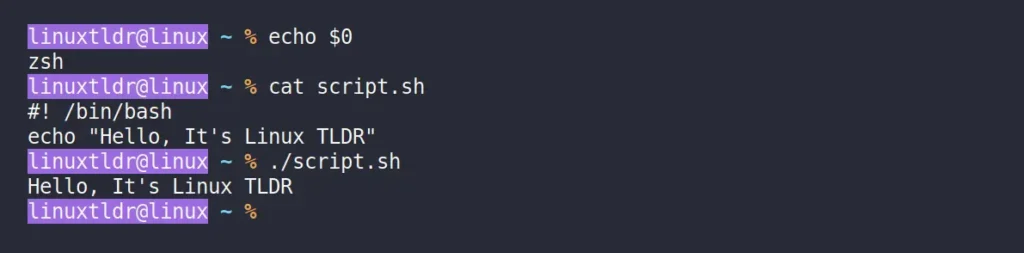
Does one always use Shebang? If you don’t specify the Shebang and run your shell script, then it will be executed from the default shell interpreter on that Linux machine.
Running a shell script from a different shell interpreter often won’t affect users much, as most shell interpreters today share a common syntax. However, there could be slight differences; for example, in ZSH, the array index starts at 1, whereas in bash, it starts at 0.
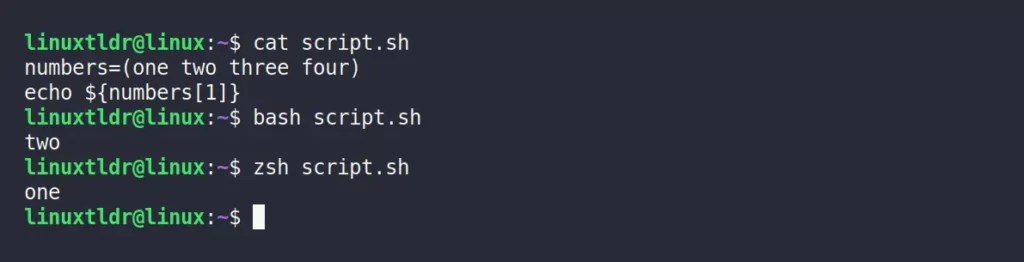
So, it is suggested to exclusively specify the shell interpreter in your shell script, and the ones that are most often specified are “sh
” and “bash
” because they are globally accepted. Even in systems where they are not set as defaults, such as Manjaro and Kali Linux, they are still present and available for users.
In most Linux distributions, a “shells
” file is maintained in the “/etc
” directory, where all the installed shell interpreter absolute paths are saved. To check it, you can use the cat or bat commands.
$ cat /etc/shells
Output:
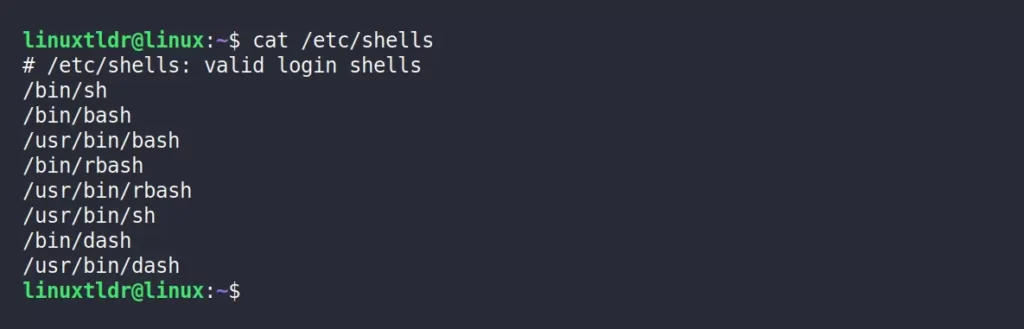
Method 5: Using the Desktop Environment File Manager (ex: Nautilus)
If you are using an Ubuntu system, you can use your file manager to run the shell script. In this case, the default shell interpreter will be used to execute the shell script.
The steps to run a shell script using the file manager are followed by:
- Open the Nautilus file manager.
- Navigate into the shell script directory.
- Right-click on the shell script and open the property window.
- Go to the Permissions tab and grant executable permission to the owner.
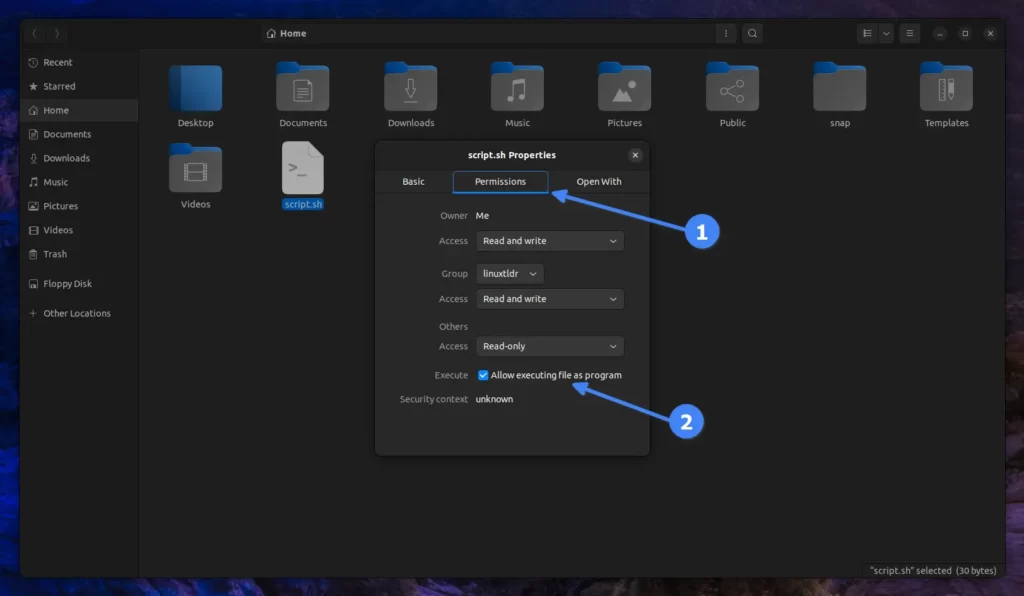
- Close the property window.
- Right-click on the shell script.
- Click on the “Run as a program” button.
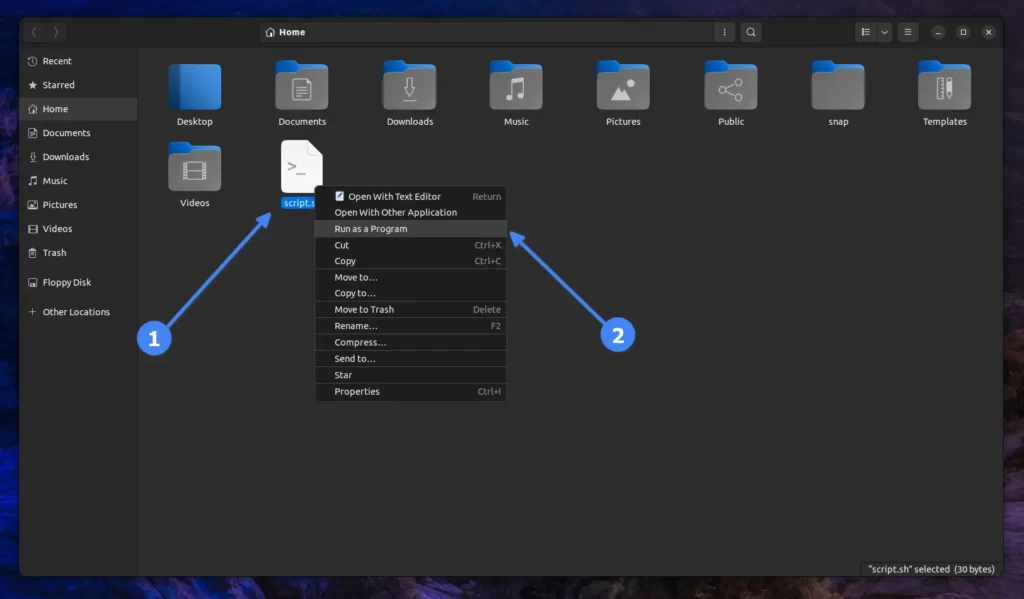
Output:
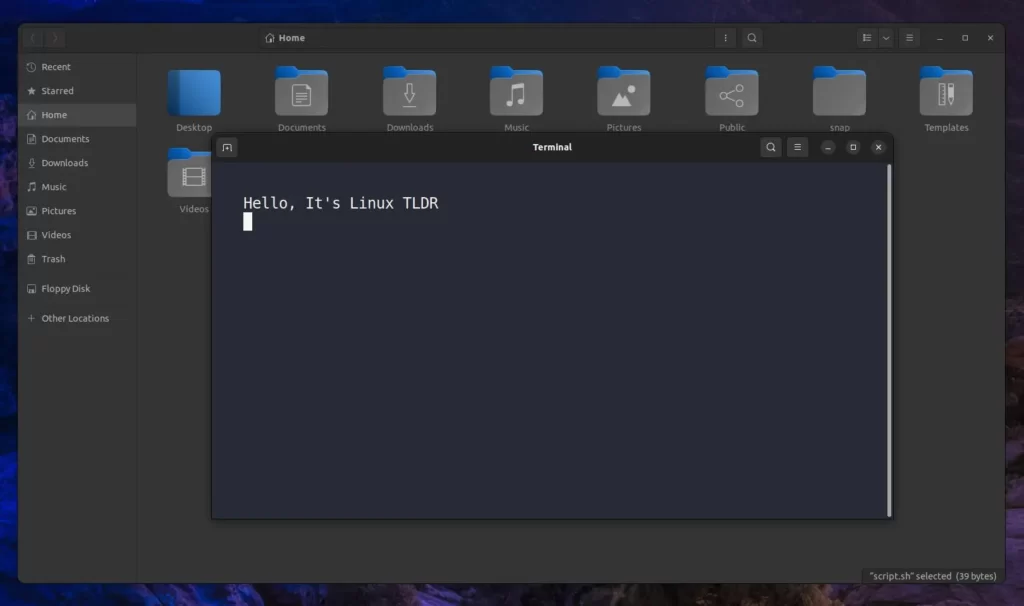
And that was the end of the article.
If you have any suggestions to include in this article, feel free to tell us in the comment section.
Till then, peace!
Just minor rant, since this is for beginners and best to set good habits at least until they understand ramifications. Best to limit handing out execute permissions, u+x instead of +x
chmod u+x